前言
TypeScript 是 JavaScript 的超集,它增加了静态类型检查和其他高级功能,目的是使代码更加健壮和可维护。它是由微软开发和维护的,最初发布于 2012 年,并得到了广泛的应用。TypeScript 在编译时会将代码转换为标准的 JavaScript,因此可以在任何支持 JavaScript 的环境中运行。
TypeScript 与java对比
特点 |
TypeScript |
Java |
编译与运行 |
编译为 JavaScript,运行于浏览器或 Node.js |
编译为字节码,运行于 JVM |
类型系统 |
静态类型检查,可选类型 |
强类型,必须显式声明类型 |
运行环境 |
前端、Node.js |
服务器端、企业级系统、安卓开发 |
面向对象支持 |
支持 OOP,类和接口为增强型 JavaScript |
纯粹的 OOP 语言 |
模块化 |
支持 ES 模块(import/export) |
Java 9 之后支持模块系统 |
调试工具 |
通过 Source Maps 调试 TypeScript 代码 |
直接调试字节码,IDE 支持完善 |
主要应用场景 |
前端开发,Node.js 后端开发 |
企业级应用、安卓开发、分布式系统 |
typescript与java语法对比
以下是 TypeScript 和 Java 在语法方面的对比表格:
功能 |
TypeScript |
Java |
变量声明 |
let , const , var |
int , double , String , boolean , char , final |
类型声明 |
number , string , boolean , void , any , unknown , enum , tuple , object |
int , double , String , boolean , char , Object , List , Map , Set |
常量 |
const PI: number = 3.14; |
static final double PI = 3.14; |
函数声明 |
function add(x: number, y: number): number { return x + y; } |
int add(int x, int y) { return x + y; } |
箭头函数 |
const multiply = (x: number, y: number): number => x * y; |
不支持箭头函数 |
类定义 |
class Person { name: string; constructor(name: string) { this.name = name; } } |
class Person { String name; Person(String name) { this.name = name; } } |
接口 |
interface Animal { name: string; } |
interface Animal { String getName(); } |
继承 |
class Dog extends Animal { breed: string; } |
class Dog extends Animal { String breed; } |
多态 |
class Animal { speak() { console.log("Animal sound"); } } class Dog extends Animal { speak() { console.log("Bark"); } } |
class Animal { void speak() { System.out.println("Animal sound"); } } class Dog extends Animal { void speak() { System.out.println("Bark"); } } |
枚举 |
enum Direction { Up, Down, Left, Right } |
public enum Direction { UP, DOWN, LEFT, RIGHT } |
泛型 |
function identity<T>(arg: T): T { return arg; } |
public <T> T identity(T arg) { return arg; } |
模块 |
export class Person {} import { Person } from './person'; |
package com.example; import com.example.Person; |
异常处理 |
try { /* code */ } catch (error) { /* handle error */ } |
try { /* code */ } catch (Exception e) { /* handle error */ } |
接口实现 |
class Dog implements Animal { name: string; constructor(name: string) { this.name = name; } } |
class Dog implements Animal { String name; } |
数据结构 |
let arr: number[] = [1, 2, 3]; let map: Map<string, number> = new Map(); |
int[] arr = {1, 2, 3}; Map<String, Integer> map = new HashMap<>(); |
类型推断 |
TypeScript 自动推断类型 |
Java 强制要求显式声明类型 |
可选参数 |
function greet(name: string, age?: number) { /* code */ } |
void greet(String name, Integer age) { /* code */ } |
默认参数 |
function greet(name: string, age: number = 30) { /* code */ } |
void greet(String name, Integer age) { if (age == null) age = 30; /* code */ } |
构造函数 |
constructor(public name: string) {} |
Person(String name) { this.name = name; } |
属性访问修饰符 |
public , private , protected |
public , private , protected |
异步操作 |
async function fetchData() { let response = await fetch(url); } |
public Future<Data> fetchData() { return CompletableFuture.supplyAsync(() -> { /* code */ }); } |
1 环境安装
1.1 npm安装
npm install -g typescript
查看版本
tsc --version
1.2 pycharm配置
安装
npm install typescript ts-node --save-dev
项目通用配置:tsconfig.json
{
"compilerOptions": {
"module": "commonjs",
"target": "es6",
"strict": true,
"esModuleInterop": true
},
"include": ["src/**/*"],
"exclude": ["node_modules"]
}
原创:有勇气的牛排
https://www.couragesteak.com/article/477
2 初始案例
hello.ts
let msg: string = "有勇气的牛排"
console.log(msg)
编译
tsc hello.ts
运行
node hello.js
3 数据类型
3.1 布尔 boolean
boolean
类型只允许 true
或 false
两个值。
let is_ban = true;
console.log(is_ban)
3.2 数值 number
number
类型用于表示所有的数字,包括整数和浮点数。
let age: number = 18;
let hex: number = 0xf00d;
let binary: number = 0b1010;
let octal: number = 0o744;
console.log(age);
console.log(hex);
console.log(binary);
console.log(octal);
3.3 字符串 string
let nickname: string = "有勇气的牛排"
let introduce: string = `${nickname}是编程大佬`
console.log(nickname);
console.log(introduce);
3.4 数组 array
tuple
允许表示一个已知数量和类型的数组,各元素的类型可以不同。
let id_list: number[] = [1, 2, 3]
let name_list: Array<string> = ['A', 'B', 'C']
console.log(id_list);
console.log(name_list);
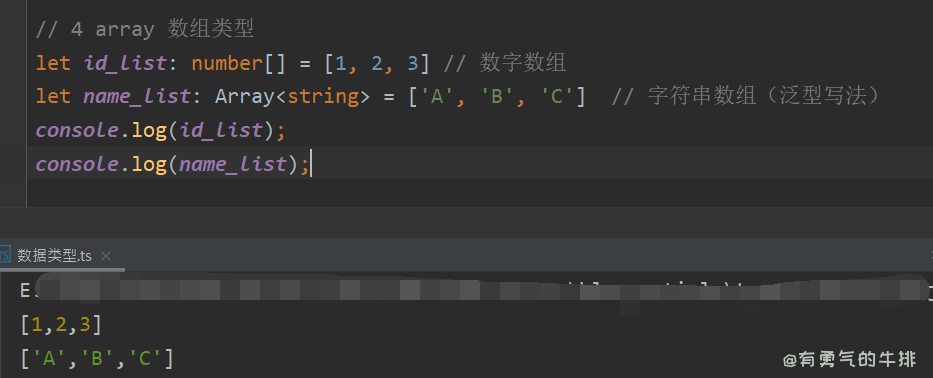
3.5 元組 tuple
let tuple: [string, number]
tuple = ["你好", 13579]
console.log(tuple);
3.6 枚举 enum
enum Color {
Red,
Green,
Blue
}
let color: Color = Color.Blue;
console.log(color);
指定开始索引
enum Direction {
Up = 1,
Down,
Left,
Right
}
console.log(Direction.Left);
3.7 任意类型 any
any
类型允许我们对某个值不确定其具体类型时使用,跳过类型检查。
let data: any = 1;
console.log(data);
data = "有勇气的牛排"
console.log(data);
data = true
console.log(data);
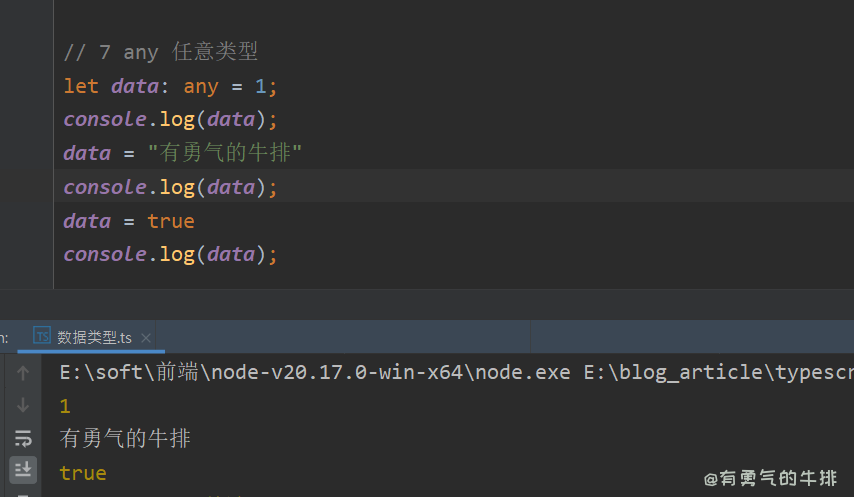
3.8 空类型 void
void
一般用于表示没有返回值的函数。它表示没有任何类型,常用于函数返回值。
function notifyMsg(): void {
console.log("有勇气的牛排");
}
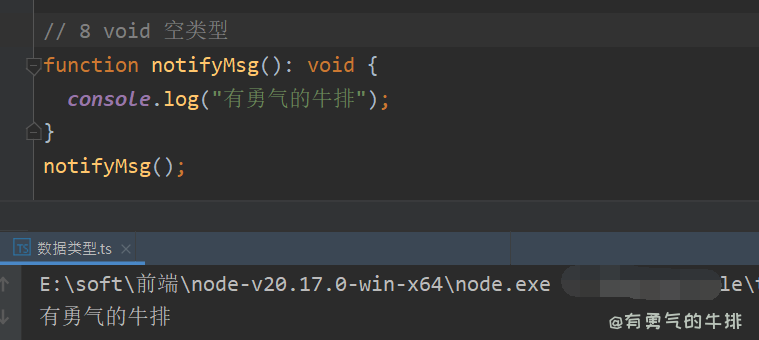
3.9 null和underfined
TypeScript 中 null
和 undefined
是所有类型的子类型。
-
null
表示空值,
-
undefined
表示未定义的值。
let id: undefined = undefined;
let name: null = null;
3.10 对象 object
object
类型表示非原始类型的对象,例如数组、函数等。
let person: object = {
id: 1,
name: "有勇气的牛排"
}
console.log(person)
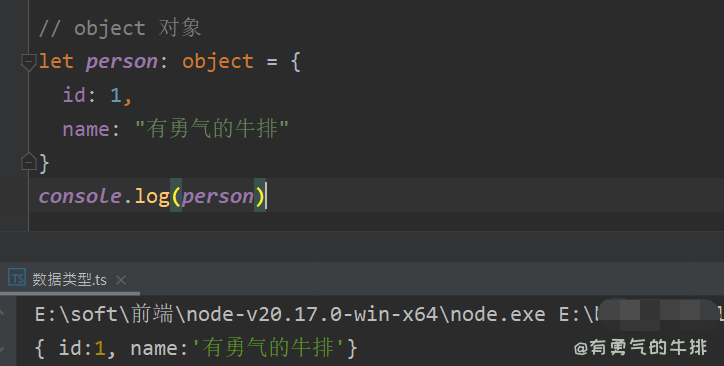
3.11 永不存在的值类型 never
function error(msg: string): never {
throw new Error(msg);
}
error("这是一个异常");
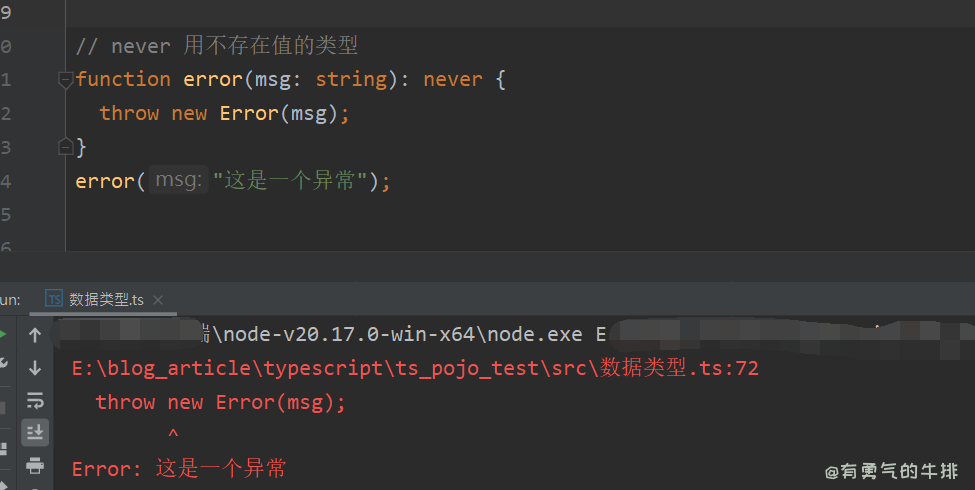
3.12 联合类型 union types
let value: string | number;
value = "有勇气的牛排"
console.log(value);
value = 1;
console.log(value);
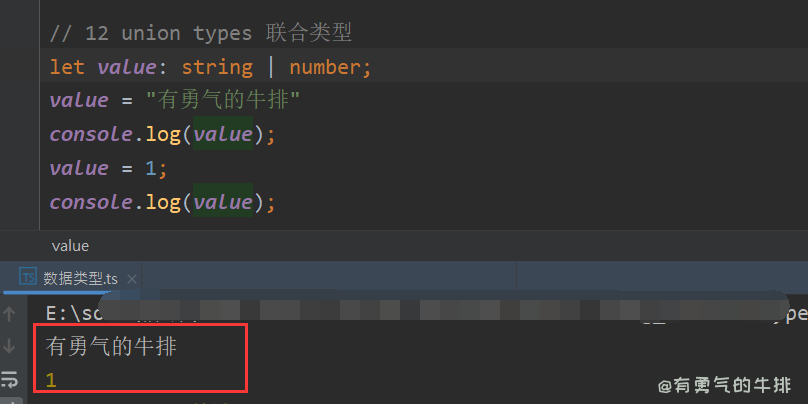
3.13 類型別名 type
type 關鍵字可以為聯合類型或複雜類型創建別名,簡化代碼
type ID = number | string;
let userId: ID;
userId = 101;
userId = "有勇气的牛排";
console.log(userId);
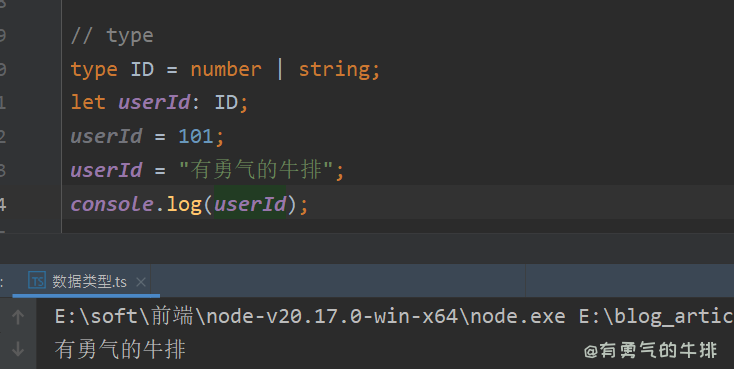
3.14 类型断言 type assertions
类型断言,允许开发者说动指定某个值的类型,类似于类型转换。
let someValue: any = "有勇气的牛排";
let strLength: number = (someValue as string).length;
console.log(strLength);
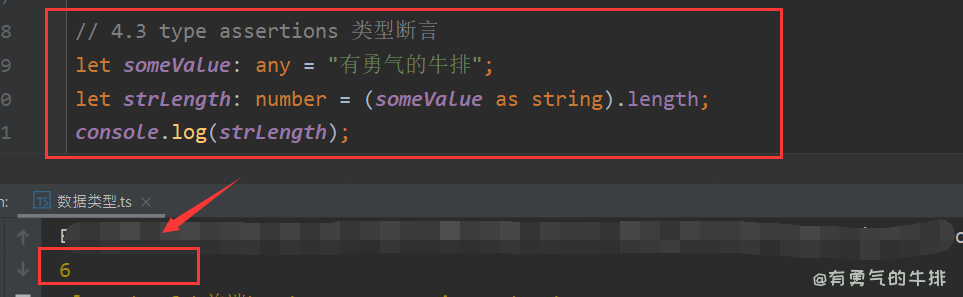
3.15 unknow
unknow 是typescript中比any更安全的类型。在不知道类型的时候可以使用。
在使用之前需要进行类型检查。
let value:unknown="有勇气的牛排Hello";
if(typeof value==='string'){
console.log(value.toUpperCase())
}
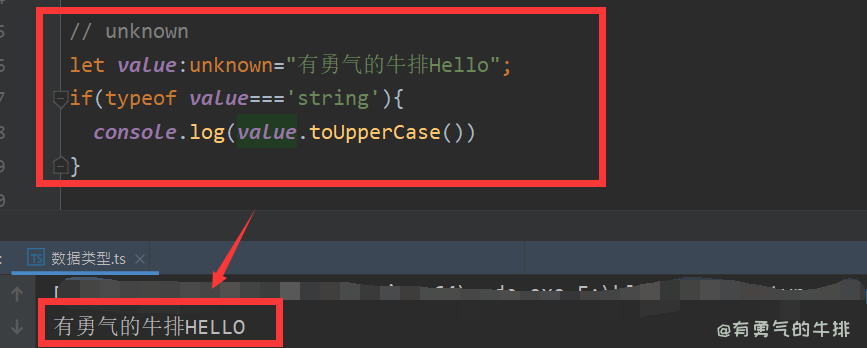
4 特殊數據類型
4.1 接口 interface
4.1.1 常规使用
interface 用於定義對象結構,描述对象包含哪些属性、属性的类型等。
interface Person {
id: number,
name: string,
}
let cs: Person = {
id: 1,
name: "有勇气的牛排"
}
console.log(cs.name)
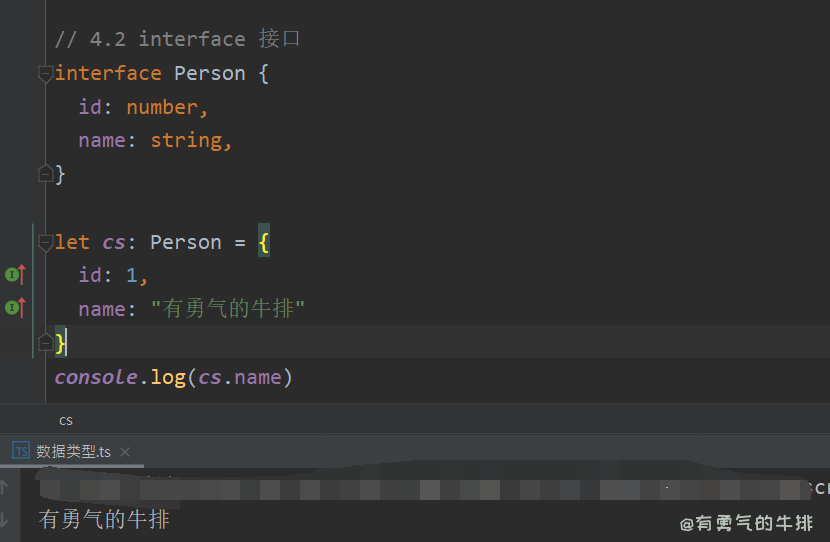
4.1.2 接口readonly
readonly 修饰符用于定义只读属性,防止在初始化后修改该属性。
interface People {
readonly id: number;
name: string;
}
let cs: People = {
id: 2,
name: "测试"
}
cs.id = 3;
console.log(cs);
4.1.3 接口可选属性 optional properties
在接口中定义可选属性,可以使用 ? 标记某个属性
interface People {
id: number;
name: string;
role?: string;
}
let cs: People = {
id: 1,
name: "有勇气的牛排",
}
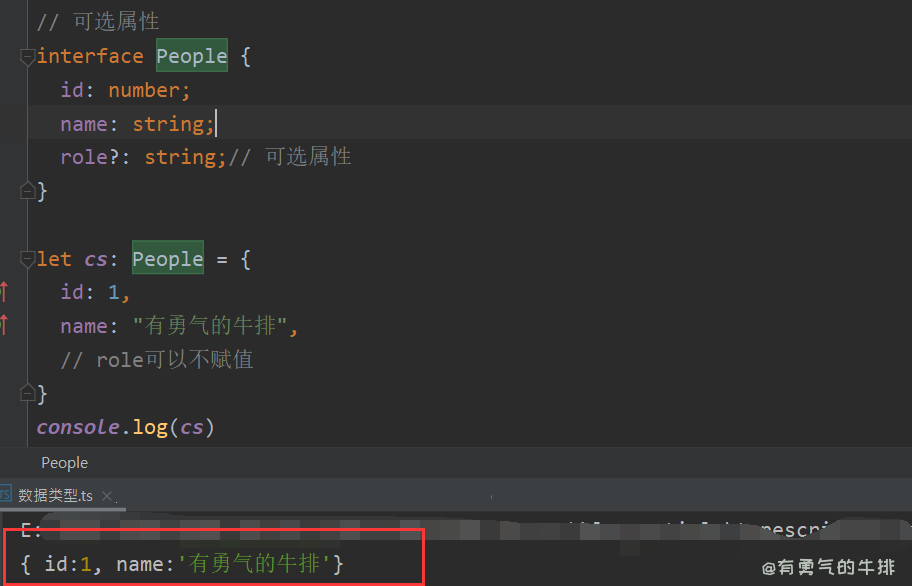
4.2 函数 function
function add(a: number, b: number): number {
return a+b;
}
console.log(add(1,2));
4.3 类
class Person {
id: number;
name: string;
constructor(id: number, name: string) {
this.id = id;
this.name = name;
}
introduce() {
console.log(`${this.name}的id为:${this.id}`)
}
}
let cs: Person = new Person(1, "有勇气的牛排");
cs.introduce();
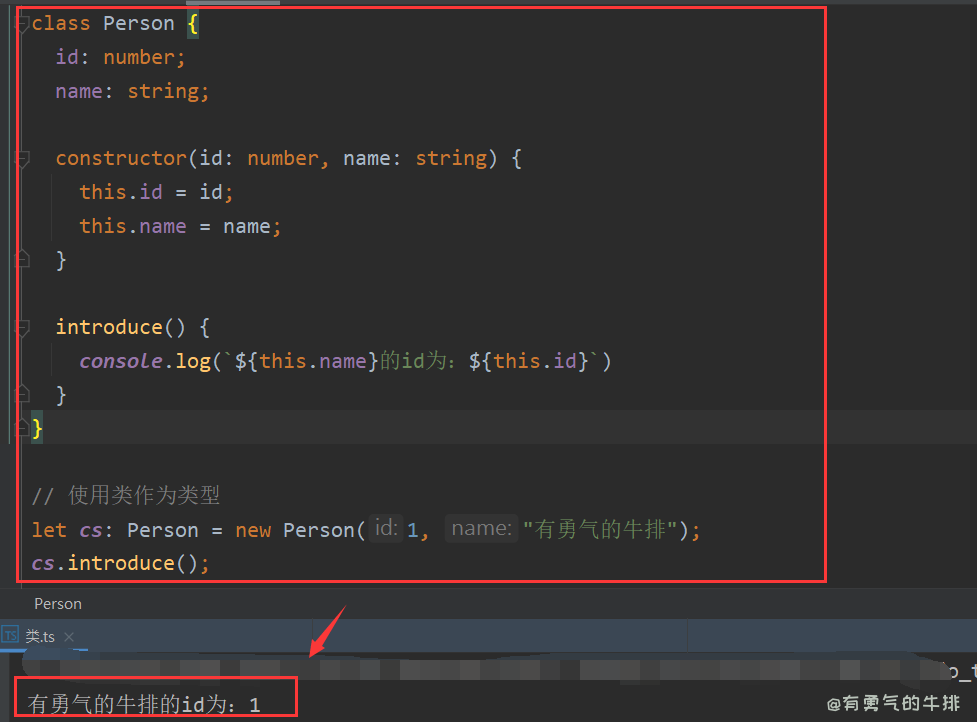
4.4 类实现接口
interface PersonInterface {
id: number;
name: string;
introduce(): void;
}
class Person implements PersonInterface{
id: number;
name: string;
constructor(id: number, name: string) {
this.id = id;
this.name = name;
}
introduce() {
console.log(`${this.name}的id为:${this.id}`)
}
}
let cs: Person = new Person(1, "有勇气的牛排");
cs.introduce();
4.5 泛型
4.5.1 泛型函数
function identity<T>(arg: T): T {
return arg;
}
let res1 = identity<string>("有勇气的牛排");
let res2 = identity<number>(100);
console.log(res1);
console.log(res2);
5 模块化开发
获取日期案例
util_time.ts
export class UtilTime {
getCurrentDate(): string {
const today = new Date();
const year = today.getFullYear();
const month = (today.getMonth() + 1).toString().padStart(2, "0");
const day = today.getDate().toString().padStart(2, "0");
const hours = today.getHours().toString().padStart(2, "0");
const minutes = today.getMinutes().toString().padStart(2, "0");
const seconds = today.getSeconds().toString().padStart(2, "0");
return `${year}年${month}月${day}日 ${hours}:${minutes}:${seconds}`;
}
getDateYesterday(days: number): string {
const date = new Date();
date.setDate(date.getDate() + days);
const year = date.getFullYear();
const month = (date.getMonth() + 1).toString().padStart(2, "0");
const day = date.getDate().toString().padStart(2, "0");
const hours = date.getHours().toString().padStart(2, "0");
const minutes = date.getMinutes().toString().padStart(2, "0");
const seconds = date.getSeconds().toString().padStart(2, "0");
return `${year}年${month}月${day}日 ${hours}:${minutes}:${seconds}`;
}
}
main.ts
import { UtilTime } from './util_time';
const util_time = new UtilTime();
const currentDate = util_time.getCurrentDate();
console.log("当前日期:", currentDate);
const daysBefore = -1;
const dateBefore = util_time.getDateYesterday(daysBefore);
console.log(`昨天日期:`, dateBefore);
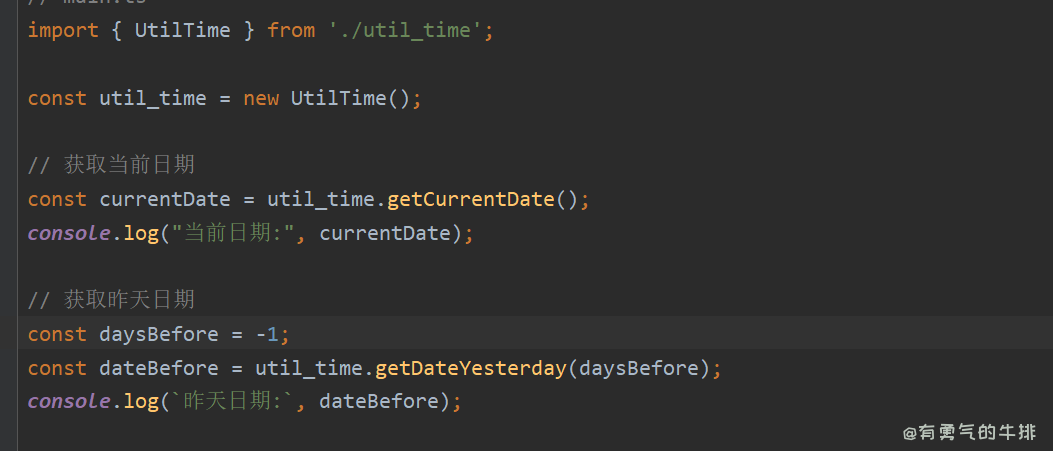
<h2><a id="_0"></a>前言</h2>
<p>TypeScript 是 JavaScript 的超集,它增加了静态类型检查和其他高级功能,目的是使代码更加健壮和可维护。它是由微软开发和维护的,最初发布于 2012 年,并得到了广泛的应用。TypeScript 在编译时会将代码转换为标准的 JavaScript,因此可以在任何支持 JavaScript 的环境中运行。</p>
<p>TypeScript 与java对比</p>
<table>
<thead>
<tr>
<th>特点</th>
<th>TypeScript</th>
<th>Java</th>
</tr>
</thead>
<tbody>
<tr>
<td>编译与运行</td>
<td>编译为 JavaScript,运行于浏览器或 Node.js</td>
<td>编译为字节码,运行于 JVM</td>
</tr>
<tr>
<td>类型系统</td>
<td>静态类型检查,可选类型</td>
<td>强类型,必须显式声明类型</td>
</tr>
<tr>
<td>运行环境</td>
<td>前端、Node.js</td>
<td>服务器端、企业级系统、安卓开发</td>
</tr>
<tr>
<td>面向对象支持</td>
<td>支持 OOP,类和接口为增强型 JavaScript</td>
<td>纯粹的 OOP 语言</td>
</tr>
<tr>
<td>模块化</td>
<td>支持 ES 模块(import/export)</td>
<td>Java 9 之后支持模块系统</td>
</tr>
<tr>
<td>调试工具</td>
<td>通过 Source Maps 调试 TypeScript 代码</td>
<td>直接调试字节码,IDE 支持完善</td>
</tr>
<tr>
<td>主要应用场景</td>
<td>前端开发,Node.js 后端开发</td>
<td>企业级应用、安卓开发、分布式系统</td>
</tr>
</tbody>
</table>
<p>typescript与java语法对比</p>
<p>以下是 TypeScript 和 Java 在语法方面的对比表格:</p>
<table>
<thead>
<tr>
<th><strong>功能</strong></th>
<th><strong>TypeScript</strong></th>
<th><strong>Java</strong></th>
</tr>
</thead>
<tbody>
<tr>
<td><strong>变量声明</strong></td>
<td><code>let</code>, <code>const</code>, <code>var</code></td>
<td><code>int</code>, <code>double</code>, <code>String</code>, <code>boolean</code>, <code>char</code>, <code>final</code></td>
</tr>
<tr>
<td><strong>类型声明</strong></td>
<td><code>number</code>, <code>string</code>, <code>boolean</code>, <code>void</code>, <code>any</code>, <code>unknown</code>, <code>enum</code>, <code>tuple</code>, <code>object</code></td>
<td><code>int</code>, <code>double</code>, <code>String</code>, <code>boolean</code>, <code>char</code>, <code>Object</code>, <code>List</code>, <code>Map</code>, <code>Set</code></td>
</tr>
<tr>
<td><strong>常量</strong></td>
<td><code>const PI: number = 3.14;</code></td>
<td><code>static final double PI = 3.14;</code></td>
</tr>
<tr>
<td><strong>函数声明</strong></td>
<td><code>function add(x: number, y: number): number { return x + y; }</code></td>
<td><code>int add(int x, int y) { return x + y; }</code></td>
</tr>
<tr>
<td><strong>箭头函数</strong></td>
<td><code>const multiply = (x: number, y: number): number => x * y;</code></td>
<td>不支持箭头函数</td>
</tr>
<tr>
<td><strong>类定义</strong></td>
<td><code>class Person { name: string; constructor(name: string) { this.name = name; } }</code></td>
<td><code>class Person { String name; Person(String name) { this.name = name; } }</code></td>
</tr>
<tr>
<td><strong>接口</strong></td>
<td><code>interface Animal { name: string; }</code></td>
<td><code>interface Animal { String getName(); }</code></td>
</tr>
<tr>
<td><strong>继承</strong></td>
<td><code>class Dog extends Animal { breed: string; }</code></td>
<td><code>class Dog extends Animal { String breed; }</code></td>
</tr>
<tr>
<td><strong>多态</strong></td>
<td><code>class Animal { speak() { console.log("Animal sound"); } }</code> <code>class Dog extends Animal { speak() { console.log("Bark"); } }</code></td>
<td><code>class Animal { void speak() { System.out.println("Animal sound"); } }</code> <code>class Dog extends Animal { void speak() { System.out.println("Bark"); } }</code></td>
</tr>
<tr>
<td><strong>枚举</strong></td>
<td><code>enum Direction { Up, Down, Left, Right }</code></td>
<td><code>public enum Direction { UP, DOWN, LEFT, RIGHT }</code></td>
</tr>
<tr>
<td><strong>泛型</strong></td>
<td><code>function identity<T>(arg: T): T { return arg; }</code></td>
<td><code>public <T> T identity(T arg) { return arg; }</code></td>
</tr>
<tr>
<td><strong>模块</strong></td>
<td><code>export class Person {}</code> <code>import { Person } from './person';</code></td>
<td><code>package com.example;</code> <code>import com.example.Person;</code></td>
</tr>
<tr>
<td><strong>异常处理</strong></td>
<td><code>try { /* code */ } catch (error) { /* handle error */ }</code></td>
<td><code>try { /* code */ } catch (Exception e) { /* handle error */ }</code></td>
</tr>
<tr>
<td><strong>接口实现</strong></td>
<td><code>class Dog implements Animal { name: string; constructor(name: string) { this.name = name; } }</code></td>
<td><code>class Dog implements Animal { String name; }</code></td>
</tr>
<tr>
<td><strong>数据结构</strong></td>
<td><code>let arr: number[] = [1, 2, 3];</code> <code>let map: Map<string, number> = new Map();</code></td>
<td><code>int[] arr = {1, 2, 3};</code> <code>Map<String, Integer> map = new HashMap<>();</code></td>
</tr>
<tr>
<td><strong>类型推断</strong></td>
<td>TypeScript 自动推断类型</td>
<td>Java 强制要求显式声明类型</td>
</tr>
<tr>
<td><strong>可选参数</strong></td>
<td><code>function greet(name: string, age?: number) { /* code */ }</code></td>
<td><code>void greet(String name, Integer age) { /* code */ }</code></td>
</tr>
<tr>
<td><strong>默认参数</strong></td>
<td><code>function greet(name: string, age: number = 30) { /* code */ }</code></td>
<td><code>void greet(String name, Integer age) { if (age == null) age = 30; /* code */ }</code></td>
</tr>
<tr>
<td><strong>构造函数</strong></td>
<td><code>constructor(public name: string) {}</code></td>
<td><code>Person(String name) { this.name = name; }</code></td>
</tr>
<tr>
<td><strong>属性访问修饰符</strong></td>
<td><code>public</code>, <code>private</code>, <code>protected</code></td>
<td><code>public</code>, <code>private</code>, <code>protected</code></td>
</tr>
<tr>
<td><strong>异步操作</strong></td>
<td><code>async function fetchData() { let response = await fetch(url); }</code></td>
<td><code>public Future<Data> fetchData() { return CompletableFuture.supplyAsync(() -> { /* code */ }); }</code></td>
</tr>
</tbody>
</table>
<h2><a id="1__44"></a>1 环境安装</h2>
<h3><a id="11_npm_46"></a>1.1 npm安装</h3>
<pre><div class="hljs"><code class="lang-shell">npm install -g typescript
</code></div></pre>
<p>查看版本</p>
<pre><div class="hljs"><code class="lang-shell">tsc --version
</code></div></pre>
<h3><a id="12_pycharm_58"></a>1.2 pycharm配置</h3>
<p>安装</p>
<pre><div class="hljs"><code class="lang-shell">npm install typescript ts-node --save-dev
</code></div></pre>
<p>项目通用配置:<code>tsconfig.json</code></p>
<pre><div class="hljs"><code class="lang-tsx">{
<span class="hljs-string">"compilerOptions"</span>: {
<span class="hljs-string">"module"</span>: <span class="hljs-string">"commonjs"</span>,
<span class="hljs-string">"target"</span>: <span class="hljs-string">"es6"</span>,
<span class="hljs-string">"strict"</span>: <span class="hljs-literal">true</span>,
<span class="hljs-string">"esModuleInterop"</span>: <span class="hljs-literal">true</span>
},
<span class="hljs-string">"include"</span>: [<span class="hljs-string">"src/**/*"</span>],
<span class="hljs-string">"exclude"</span>: [<span class="hljs-string">"node_modules"</span>]
}
</code></div></pre>
<p>原创:有勇气的牛排<br />
<a href="https://www.couragesteak.com/article/477" target="_blank">https://www.couragesteak.com/article/477</a></p>
<h2><a id="2__84"></a>2 初始案例</h2>
<p>hello.ts</p>
<pre><div class="hljs"><code class="lang-shell">let msg: string = "有勇气的牛排"
console.log(msg)
</code></div></pre>
<p>编译</p>
<pre><div class="hljs"><code class="lang-shell">tsc hello.ts
</code></div></pre>
<p>运行</p>
<pre><div class="hljs"><code class="lang-shell">node hello.js
</code></div></pre>
<h2><a id="3__107"></a>3 数据类型</h2>
<h3><a id="31__boolean_109"></a>3.1 布尔 boolean</h3>
<p><code>boolean</code> 类型只允许 <code>true</code> 或 <code>false</code> 两个值。</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> is_ban = <span class="hljs-literal">true</span>;
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(is_ban)
</code></div></pre>
<h3><a id="32__number_118"></a>3.2 数值 number</h3>
<p><code>number</code> 类型用于表示所有的数字,包括整数和浮点数。</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> <span class="hljs-attr">age</span>: <span class="hljs-built_in">number</span> = <span class="hljs-number">18</span>;
<span class="hljs-keyword">let</span> <span class="hljs-attr">hex</span>: <span class="hljs-built_in">number</span> = <span class="hljs-number">0xf00d</span>; <span class="hljs-comment">//16进制</span>
<span class="hljs-keyword">let</span> <span class="hljs-attr">binary</span>: <span class="hljs-built_in">number</span> = <span class="hljs-number">0b1010</span>; <span class="hljs-comment">//2进制</span>
<span class="hljs-keyword">let</span> <span class="hljs-attr">octal</span>: <span class="hljs-built_in">number</span> = <span class="hljs-number">0o744</span>; <span class="hljs-comment">// 8进制</span>
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(age);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(hex);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(binary);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(octal);
</code></div></pre>
<h3><a id="33__string_133"></a>3.3 字符串 string</h3>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> <span class="hljs-attr">nickname</span>: <span class="hljs-built_in">string</span> = <span class="hljs-string">"有勇气的牛排"</span>
<span class="hljs-keyword">let</span> <span class="hljs-attr">introduce</span>: <span class="hljs-built_in">string</span> = <span class="hljs-string">`<span class="hljs-subst">${nickname}</span>是编程大佬`</span>
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(nickname);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(introduce);
</code></div></pre>
<h3><a id="34__array_142"></a>3.4 数组 array</h3>
<p><code>tuple</code> 允许表示一个已知数量和类型的数组,各元素的类型可以不同。</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> <span class="hljs-attr">id_list</span>: <span class="hljs-built_in">number</span>[] = [<span class="hljs-number">1</span>, <span class="hljs-number">2</span>, <span class="hljs-number">3</span>] <span class="hljs-comment">// 数字数组</span>
<span class="hljs-keyword">let</span> <span class="hljs-attr">name_list</span>: <span class="hljs-title class_">Array</span><<span class="hljs-built_in">string</span>> = [<span class="hljs-string">'A'</span>, <span class="hljs-string">'B'</span>, <span class="hljs-string">'C'</span>] <span class="hljs-comment">// 字符串数组(泛型写法)</span>
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(id_list);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(name_list);
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/fc5c743b0d32f64d335edc7bcac1046f.png" alt="image.png" /></p>
<h3><a id="35__tuple_155"></a>3.5 元組 tuple</h3>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> <span class="hljs-attr">tuple</span>: [<span class="hljs-built_in">string</span>, <span class="hljs-built_in">number</span>]
tuple = [<span class="hljs-string">"你好"</span>, <span class="hljs-number">13579</span>]
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(tuple);
</code></div></pre>
<h3><a id="36__enum_167"></a>3.6 枚举 enum</h3>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-built_in">enum</span> <span class="hljs-title class_">Color</span> {
<span class="hljs-title class_">Red</span>,
<span class="hljs-title class_">Green</span>,
<span class="hljs-title class_">Blue</span>
}
<span class="hljs-keyword">let</span> <span class="hljs-attr">color</span>: <span class="hljs-title class_">Color</span> = <span class="hljs-title class_">Color</span>.<span class="hljs-property">Blue</span>;
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(color);
</code></div></pre>
<p>指定开始索引</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-built_in">enum</span> <span class="hljs-title class_">Direction</span> {
<span class="hljs-title class_">Up</span> = <span class="hljs-number">1</span>,
<span class="hljs-title class_">Down</span>,
<span class="hljs-title class_">Left</span>,
<span class="hljs-title class_">Right</span>
}
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-title class_">Direction</span>.<span class="hljs-property">Left</span>);
</code></div></pre>
<h3><a id="37__any_193"></a>3.7 任意类型 any</h3>
<p><code>any</code> 类型允许我们对某个值不确定其具体类型时使用,跳过类型检查。</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> <span class="hljs-attr">data</span>: <span class="hljs-built_in">any</span> = <span class="hljs-number">1</span>;
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(data);
data = <span class="hljs-string">"有勇气的牛排"</span>
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(data);
data = <span class="hljs-literal">true</span>
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(data);
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/026ae56916fedca31996a1f77282d82d.png" alt="image.png" /></p>
<h3><a id="38__void_210"></a>3.8 空类型 void</h3>
<p><code>void</code> 一般用于表示没有返回值的函数。它表示没有任何类型,常用于函数返回值。</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">function</span> <span class="hljs-title function_">notifyMsg</span>(<span class="hljs-params"></span>): <span class="hljs-built_in">void</span> {
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">"有勇气的牛排"</span>);
}
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/e9967955f0dda084b07a4bfe42234386.png" alt="image.png" /></p>
<h3><a id="39_nullunderfined_224"></a>3.9 null和underfined</h3>
<p>TypeScript 中 <code>null</code> 和 <code>undefined</code> 是所有类型的子类型。</p>
<ul>
<li>
<p><code>null</code> 表示空值,</p>
</li>
<li>
<p><code>undefined</code> 表示未定义的值。</p>
</li>
</ul>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> <span class="hljs-attr">id</span>: <span class="hljs-literal">undefined</span> = <span class="hljs-literal">undefined</span>;
<span class="hljs-keyword">let</span> <span class="hljs-attr">name</span>: <span class="hljs-literal">null</span> = <span class="hljs-literal">null</span>;
</code></div></pre>
<h3><a id="310__object_237"></a>3.10 对象 object</h3>
<p><code>object</code> 类型表示非原始类型的对象,例如数组、函数等。</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> <span class="hljs-attr">person</span>: <span class="hljs-built_in">object</span> = {
<span class="hljs-attr">id</span>: <span class="hljs-number">1</span>,
<span class="hljs-attr">name</span>: <span class="hljs-string">"有勇气的牛排"</span>
}
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(person)
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/9ec089087c757ac89f1267712a7c21bc.png" alt="image.png" /></p>
<h3><a id="311__never_255"></a>3.11 永不存在的值类型 never</h3>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">function</span> <span class="hljs-title function_">error</span>(<span class="hljs-params">msg: <span class="hljs-built_in">string</span></span>): <span class="hljs-built_in">never</span> {
<span class="hljs-keyword">throw</span> <span class="hljs-keyword">new</span> <span class="hljs-title class_">Error</span>(msg);
}
<span class="hljs-title function_">error</span>(<span class="hljs-string">"这是一个异常"</span>);
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/6d79f1f7c557f5f2fd3a38dad6296960.png" alt="image.png" /></p>
<h3><a id="312__union_types_266"></a>3.12 联合类型 union types</h3>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> <span class="hljs-attr">value</span>: <span class="hljs-built_in">string</span> | <span class="hljs-built_in">number</span>;
value = <span class="hljs-string">"有勇气的牛排"</span>
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(value);
value = <span class="hljs-number">1</span>;
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(value);
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/ea8fa745c78971db5b9864a487bab5cb.png" alt="image.png" /></p>
<h3><a id="313__type_278"></a>3.13 類型別名 type</h3>
<p>type 關鍵字可以為<strong>聯合類型</strong>或<strong>複雜類型</strong>創建<strong>別名</strong>,簡化代碼</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">type</span> <span class="hljs-variable constant_">ID</span> = <span class="hljs-built_in">number</span> | <span class="hljs-built_in">string</span>;
<span class="hljs-keyword">let</span> <span class="hljs-attr">userId</span>: <span class="hljs-variable constant_">ID</span>;
userId = <span class="hljs-number">101</span>;
userId = <span class="hljs-string">"有勇气的牛排"</span>;
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(userId);
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/1b3fc72ef2a02c521aaa877aa4343ddc.png" alt="image.png" /></p>
<h3><a id="314__type_assertions_292"></a>3.14 类型断言 type assertions</h3>
<p>类型断言,允许开发者说动指定某个值的类型,类似于类型转换。</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> <span class="hljs-attr">someValue</span>: <span class="hljs-built_in">any</span> = <span class="hljs-string">"有勇气的牛排"</span>;
<span class="hljs-keyword">let</span> <span class="hljs-attr">strLength</span>: <span class="hljs-built_in">number</span> = (someValue <span class="hljs-keyword">as</span> <span class="hljs-built_in">string</span>).<span class="hljs-property">length</span>;
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(strLength);
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/b5e7bc9019dc4edf6fb3db309fd81376.png" alt="image.png" /></p>
<h3><a id="315_unknow_304"></a>3.15 unknow</h3>
<p>unknow 是typescript中比any更安全的类型。在不知道类型的时候可以使用。</p>
<p>在使用之前需要进行类型检查。</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">let</span> <span class="hljs-attr">value</span>:unknown=<span class="hljs-string">"有勇气的牛排Hello"</span>;
<span class="hljs-keyword">if</span>(<span class="hljs-keyword">typeof</span> value===<span class="hljs-string">'string'</span>){
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(value.<span class="hljs-title function_">toUpperCase</span>())
}
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/05e8b954998f83cabaf87703ddb88f5d.png" alt="image.png" /></p>
<h3><a id="_319"></a></h3>
<h2><a id="4__321"></a>4 特殊數據類型</h2>
<h3><a id="41__interface_323"></a>4.1 接口 interface</h3>
<h4><a id="411__325"></a>4.1.1 常规使用</h4>
<p>interface 用於定義對象結構,描述对象包含哪些属性、属性的类型等。</p>
<pre><div class="hljs"><code class="lang-jsx">interface <span class="hljs-title class_">Person</span> {
<span class="hljs-attr">id</span>: number,
<span class="hljs-attr">name</span>: string,
}
<span class="hljs-keyword">let</span> <span class="hljs-attr">cs</span>: <span class="hljs-title class_">Person</span> = {
<span class="hljs-attr">id</span>: <span class="hljs-number">1</span>,
<span class="hljs-attr">name</span>: <span class="hljs-string">"有勇气的牛排"</span>
}
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(cs.<span class="hljs-property">name</span>)
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/04c74e4b54f531cc094bd58297fc100e.png" alt="image.png" /></p>
<h4><a id="412_readonly_344"></a>4.1.2 接口readonly</h4>
<p>readonly 修饰符用于定义只读属性,防止在初始化后修改该属性。</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">interface</span> <span class="hljs-title class_">People</span> {
<span class="hljs-keyword">readonly</span> <span class="hljs-attr">id</span>: <span class="hljs-built_in">number</span>;
<span class="hljs-attr">name</span>: <span class="hljs-built_in">string</span>;
}
<span class="hljs-keyword">let</span> <span class="hljs-attr">cs</span>: <span class="hljs-title class_">People</span> = {
<span class="hljs-attr">id</span>: <span class="hljs-number">2</span>,
<span class="hljs-attr">name</span>: <span class="hljs-string">"测试"</span>
}
<span class="hljs-comment">// 尝试修改只读属性</span>
cs.<span class="hljs-property">id</span> = <span class="hljs-number">3</span>; <span class="hljs-comment">// 这里应该会报错,因为 id 是只读属性</span>
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(cs);
</code></div></pre>
<h4><a id="413__optional_properties_365"></a>4.1.3 接口可选属性 optional properties</h4>
<p>在接口中定义可选属性,可以使用 ? 标记某个属性</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">interface</span> <span class="hljs-title class_">People</span> {
<span class="hljs-attr">id</span>: <span class="hljs-built_in">number</span>;
<span class="hljs-attr">name</span>: <span class="hljs-built_in">string</span>;
role?: <span class="hljs-built_in">string</span>;<span class="hljs-comment">// 可选属性</span>
}
<span class="hljs-keyword">let</span> <span class="hljs-attr">cs</span>: <span class="hljs-title class_">People</span> = {
<span class="hljs-attr">id</span>: <span class="hljs-number">1</span>,
<span class="hljs-attr">name</span>: <span class="hljs-string">"有勇气的牛排"</span>,
<span class="hljs-comment">// role可以不赋值</span>
}
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/329d16edf9c2a9a672d03e948a1581a5.png" alt="image.png" /></p>
<h3><a id="42__function_385"></a>4.2 函数 function</h3>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">function</span> <span class="hljs-title function_">add</span>(<span class="hljs-params">a: <span class="hljs-built_in">number</span>, b: <span class="hljs-built_in">number</span></span>): <span class="hljs-built_in">number</span> {
<span class="hljs-keyword">return</span> a+b;
}
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-title function_">add</span>(<span class="hljs-number">1</span>,<span class="hljs-number">2</span>));
</code></div></pre>
<h3><a id="43__394"></a>4.3 类</h3>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">class</span> <span class="hljs-title class_">Person</span> {
<span class="hljs-attr">id</span>: <span class="hljs-built_in">number</span>;
<span class="hljs-attr">name</span>: <span class="hljs-built_in">string</span>;
<span class="hljs-title function_">constructor</span>(<span class="hljs-params">id: <span class="hljs-built_in">number</span>, name: <span class="hljs-built_in">string</span></span>) {
<span class="hljs-variable language_">this</span>.<span class="hljs-property">id</span> = id;
<span class="hljs-variable language_">this</span>.<span class="hljs-property">name</span> = name;
}
<span class="hljs-title function_">introduce</span>(<span class="hljs-params"></span>) {
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">`<span class="hljs-subst">${<span class="hljs-variable language_">this</span>.name}</span>的id为:<span class="hljs-subst">${<span class="hljs-variable language_">this</span>.id}</span>`</span>)
}
}
<span class="hljs-comment">// 使用类作为类型</span>
<span class="hljs-keyword">let</span> <span class="hljs-attr">cs</span>: <span class="hljs-title class_">Person</span> = <span class="hljs-keyword">new</span> <span class="hljs-title class_">Person</span>(<span class="hljs-number">1</span>, <span class="hljs-string">"有勇气的牛排"</span>);
cs.<span class="hljs-title function_">introduce</span>();
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/0bc16988e962678fb3d3facfd2bfba6d.png" alt="image.png" /></p>
<h3><a id="44__418"></a>4.4 类实现接口</h3>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">interface</span> <span class="hljs-title class_">PersonInterface</span> {
<span class="hljs-attr">id</span>: <span class="hljs-built_in">number</span>;
<span class="hljs-attr">name</span>: <span class="hljs-built_in">string</span>;
<span class="hljs-title function_">introduce</span>(): <span class="hljs-built_in">void</span>;
}
<span class="hljs-comment">// 接口扩展类的结构。也可以在接口中定义类的行为,或者让一个类实现接口。</span>
<span class="hljs-keyword">class</span> <span class="hljs-title class_">Person</span> <span class="hljs-keyword">implements</span> <span class="hljs-title class_">PersonInterface</span>{
<span class="hljs-attr">id</span>: <span class="hljs-built_in">number</span>;
<span class="hljs-attr">name</span>: <span class="hljs-built_in">string</span>;
<span class="hljs-title function_">constructor</span>(<span class="hljs-params">id: <span class="hljs-built_in">number</span>, name: <span class="hljs-built_in">string</span></span>) {
<span class="hljs-variable language_">this</span>.<span class="hljs-property">id</span> = id;
<span class="hljs-variable language_">this</span>.<span class="hljs-property">name</span> = name;
}
<span class="hljs-title function_">introduce</span>(<span class="hljs-params"></span>) {
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">`<span class="hljs-subst">${<span class="hljs-variable language_">this</span>.name}</span>的id为:<span class="hljs-subst">${<span class="hljs-variable language_">this</span>.id}</span>`</span>)
}
}
<span class="hljs-comment">// 使用类作为类型</span>
<span class="hljs-keyword">let</span> <span class="hljs-attr">cs</span>: <span class="hljs-title class_">Person</span> = <span class="hljs-keyword">new</span> <span class="hljs-title class_">Person</span>(<span class="hljs-number">1</span>, <span class="hljs-string">"有勇气的牛排"</span>);
cs.<span class="hljs-title function_">introduce</span>();
</code></div></pre>
<h3><a id="45__448"></a>4.5 泛型</h3>
<h4><a id="451__450"></a>4.5.1 泛型函数</h4>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-keyword">function</span> identity<T>(<span class="hljs-attr">arg</span>: T): T {
<span class="hljs-keyword">return</span> arg;
}
<span class="hljs-keyword">let</span> res1 = identity<<span class="hljs-built_in">string</span>>(<span class="hljs-string">"有勇气的牛排"</span>);
<span class="hljs-keyword">let</span> res2 = identity<<span class="hljs-built_in">number</span>>(<span class="hljs-number">100</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(res1);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(res2);
</code></div></pre>
<h2><a id="5__465"></a>5 模块化开发</h2>
<p>获取日期案例</p>
<p><code>util_time.ts</code></p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-comment">// DateUtils.ts</span>
<span class="hljs-keyword">export</span> <span class="hljs-keyword">class</span> <span class="hljs-title class_">UtilTime</span> {
<span class="hljs-comment">// 获取当前日期</span>
<span class="hljs-title function_">getCurrentDate</span>(): <span class="hljs-built_in">string</span> {
<span class="hljs-keyword">const</span> today = <span class="hljs-keyword">new</span> <span class="hljs-title class_">Date</span>();
<span class="hljs-keyword">const</span> year = today.<span class="hljs-title function_">getFullYear</span>();
<span class="hljs-keyword">const</span> month = (today.<span class="hljs-title function_">getMonth</span>() + <span class="hljs-number">1</span>).<span class="hljs-title function_">toString</span>().<span class="hljs-title function_">padStart</span>(<span class="hljs-number">2</span>, <span class="hljs-string">"0"</span>);
<span class="hljs-keyword">const</span> day = today.<span class="hljs-title function_">getDate</span>().<span class="hljs-title function_">toString</span>().<span class="hljs-title function_">padStart</span>(<span class="hljs-number">2</span>, <span class="hljs-string">"0"</span>);
<span class="hljs-keyword">const</span> hours = today.<span class="hljs-title function_">getHours</span>().<span class="hljs-title function_">toString</span>().<span class="hljs-title function_">padStart</span>(<span class="hljs-number">2</span>, <span class="hljs-string">"0"</span>);
<span class="hljs-keyword">const</span> minutes = today.<span class="hljs-title function_">getMinutes</span>().<span class="hljs-title function_">toString</span>().<span class="hljs-title function_">padStart</span>(<span class="hljs-number">2</span>, <span class="hljs-string">"0"</span>);
<span class="hljs-keyword">const</span> seconds = today.<span class="hljs-title function_">getSeconds</span>().<span class="hljs-title function_">toString</span>().<span class="hljs-title function_">padStart</span>(<span class="hljs-number">2</span>, <span class="hljs-string">"0"</span>);
<span class="hljs-keyword">return</span> <span class="hljs-string">`<span class="hljs-subst">${year}</span>年<span class="hljs-subst">${month}</span>月<span class="hljs-subst">${day}</span>日 <span class="hljs-subst">${hours}</span>:<span class="hljs-subst">${minutes}</span>:<span class="hljs-subst">${seconds}</span>`</span>;
}
<span class="hljs-comment">// 获取 n 天前的日期</span>
<span class="hljs-title function_">getDateYesterday</span>(<span class="hljs-attr">days</span>: <span class="hljs-built_in">number</span>): <span class="hljs-built_in">string</span> {
<span class="hljs-keyword">const</span> date = <span class="hljs-keyword">new</span> <span class="hljs-title class_">Date</span>();
date.<span class="hljs-title function_">setDate</span>(date.<span class="hljs-title function_">getDate</span>() + days); <span class="hljs-comment">// 设置为 n 天前</span>
<span class="hljs-keyword">const</span> year = date.<span class="hljs-title function_">getFullYear</span>();
<span class="hljs-keyword">const</span> month = (date.<span class="hljs-title function_">getMonth</span>() + <span class="hljs-number">1</span>).<span class="hljs-title function_">toString</span>().<span class="hljs-title function_">padStart</span>(<span class="hljs-number">2</span>, <span class="hljs-string">"0"</span>);
<span class="hljs-keyword">const</span> day = date.<span class="hljs-title function_">getDate</span>().<span class="hljs-title function_">toString</span>().<span class="hljs-title function_">padStart</span>(<span class="hljs-number">2</span>, <span class="hljs-string">"0"</span>);
<span class="hljs-keyword">const</span> hours = date.<span class="hljs-title function_">getHours</span>().<span class="hljs-title function_">toString</span>().<span class="hljs-title function_">padStart</span>(<span class="hljs-number">2</span>, <span class="hljs-string">"0"</span>);
<span class="hljs-keyword">const</span> minutes = date.<span class="hljs-title function_">getMinutes</span>().<span class="hljs-title function_">toString</span>().<span class="hljs-title function_">padStart</span>(<span class="hljs-number">2</span>, <span class="hljs-string">"0"</span>);
<span class="hljs-keyword">const</span> seconds = date.<span class="hljs-title function_">getSeconds</span>().<span class="hljs-title function_">toString</span>().<span class="hljs-title function_">padStart</span>(<span class="hljs-number">2</span>, <span class="hljs-string">"0"</span>);
<span class="hljs-keyword">return</span> <span class="hljs-string">`<span class="hljs-subst">${year}</span>年<span class="hljs-subst">${month}</span>月<span class="hljs-subst">${day}</span>日 <span class="hljs-subst">${hours}</span>:<span class="hljs-subst">${minutes}</span>:<span class="hljs-subst">${seconds}</span>`</span>;
}
}
</code></div></pre>
<p><code>main.ts</code></p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-comment">// main.ts</span>
<span class="hljs-keyword">import</span> { <span class="hljs-title class_">UtilTime</span> } <span class="hljs-keyword">from</span> <span class="hljs-string">'./util_time'</span>;
<span class="hljs-keyword">const</span> util_time = <span class="hljs-keyword">new</span> <span class="hljs-title class_">UtilTime</span>();
<span class="hljs-comment">// 获取当前日期</span>
<span class="hljs-keyword">const</span> currentDate = util_time.<span class="hljs-title function_">getCurrentDate</span>();
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">"当前日期:"</span>, currentDate);
<span class="hljs-comment">// 获取昨天日期</span>
<span class="hljs-keyword">const</span> daysBefore = -<span class="hljs-number">1</span>;
<span class="hljs-keyword">const</span> dateBefore = util_time.<span class="hljs-title function_">getDateYesterday</span>(daysBefore);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">`昨天日期:`</span>, dateBefore);
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/2ef63262a80c063c7b381a8ed4b77a32.png" alt="image.png" /></p>
留言