1 GET 参数
router.get('/get_test', async (req: Request, res: Response) => {
const {name, id} = req.query;
const data = {id, name}
ResponseUtil.success(res, data, "查询成功", 20000);
});

2 POST 参数
http://127.0.0.1:3000/api/v1/post_json
json请求体
{
"username": "cs",
"password": "123456"
}
后端
router.post('/post_json', async (req: Request, res: Response) => {
const { username, password } = req.body;
const data = { username, password }
ResponseUtil.success(res, data, "查询成功", 20000);
});
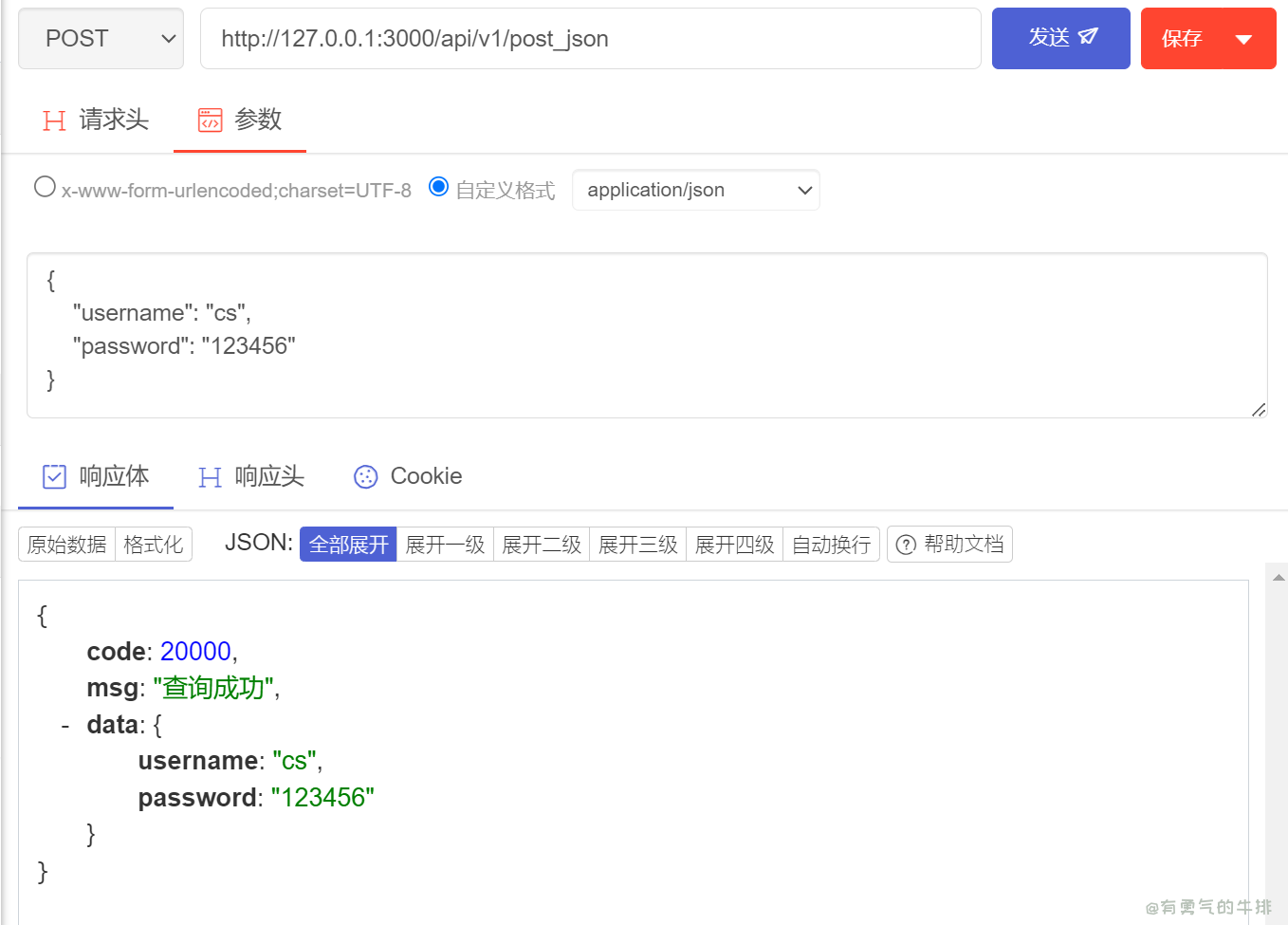
3 动态路由参数
http://127.0.0.1:3000/api/v1/user/1
router.get('/user/:id', async (req: Request, res: Response) => {
const { id } = req.params;
const data = { id }
ResponseUtil.success(res, data, "查询成功", 20000);
});
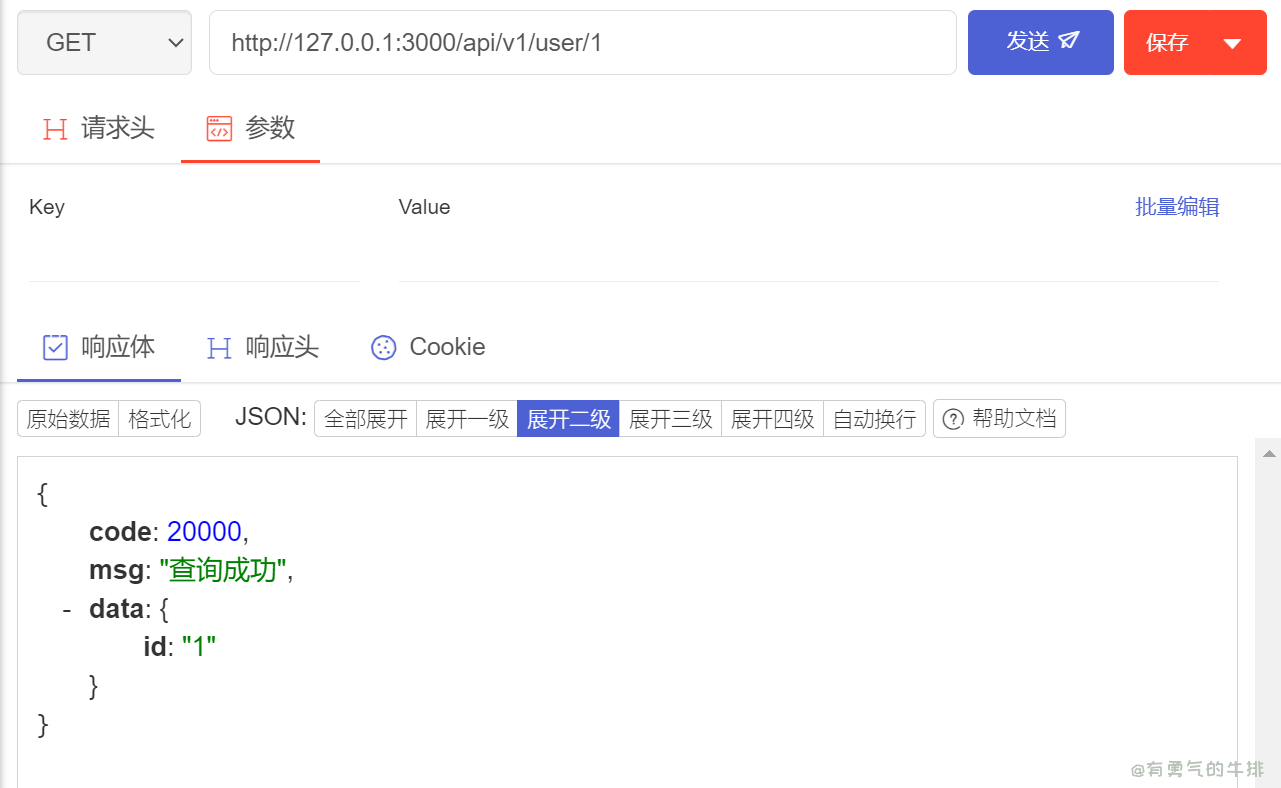
<h2><a id="1_GET__0"></a>1 GET 参数</h2>
<pre><div class="hljs"><code class="lang-ts"><span class="hljs-comment">// GET 参数测试</span>
<span class="hljs-comment">// http://localhost:3000/api/v1/get_test?name=有勇气的牛排&id=1</span>
router.<span class="hljs-title function_">get</span>(<span class="hljs-string">'/get_test'</span>, <span class="hljs-keyword">async</span> (<span class="hljs-attr">req</span>: <span class="hljs-title class_">Request</span>, <span class="hljs-attr">res</span>: <span class="hljs-title class_">Response</span>) => {
<span class="hljs-keyword">const</span> {name, id} = req.<span class="hljs-property">query</span>; <span class="hljs-comment">// 从查询字符串中提取参数</span>
<span class="hljs-keyword">const</span> data = {id, name}
<span class="hljs-title class_">ResponseUtil</span>.<span class="hljs-title function_">success</span>(res, data, <span class="hljs-string">"查询成功"</span>, <span class="hljs-number">20000</span>);
});
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/f325da3658db1c25e846fb376236f1fc.png" alt="image.png" /></p>
<h2><a id="2_POST__14"></a>2 POST 参数</h2>
<p>http://127.0.0.1:3000/api/v1/post_json</p>
<p>json请求体</p>
<pre><div class="hljs"><code class="lang-json"><span class="hljs-punctuation">{</span>
<span class="hljs-attr">"username"</span><span class="hljs-punctuation">:</span> <span class="hljs-string">"cs"</span><span class="hljs-punctuation">,</span>
<span class="hljs-attr">"password"</span><span class="hljs-punctuation">:</span> <span class="hljs-string">"123456"</span>
<span class="hljs-punctuation">}</span>
</code></div></pre>
<p>后端</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-comment">// POST_JSON 参数测试</span>
<span class="hljs-comment">// http://127.0.0.1:3000/api/v1/post_json</span>
router.<span class="hljs-title function_">post</span>(<span class="hljs-string">'/post_json'</span>, <span class="hljs-keyword">async</span> (<span class="hljs-attr">req</span>: <span class="hljs-title class_">Request</span>, <span class="hljs-attr">res</span>: <span class="hljs-title class_">Response</span>) => {
<span class="hljs-keyword">const</span> { username, password } = req.<span class="hljs-property">body</span>; <span class="hljs-comment">// 从请求体中提取参数</span>
<span class="hljs-keyword">const</span> data = { username, password }
<span class="hljs-title class_">ResponseUtil</span>.<span class="hljs-title function_">success</span>(res, data, <span class="hljs-string">"查询成功"</span>, <span class="hljs-number">20000</span>);
});
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/0caedc281096010b979c79e108ee74ad.png" alt="image.png" /></p>
<h2><a id="3__41"></a>3 动态路由参数</h2>
<p>http://127.0.0.1:3000/api/v1/user/1</p>
<pre><div class="hljs"><code class="lang-tsx"><span class="hljs-comment">// 动态路由参数</span>
<span class="hljs-comment">// http://127.0.0.1:3000/api/v1/user/1</span>
router.<span class="hljs-title function_">get</span>(<span class="hljs-string">'/user/:id'</span>, <span class="hljs-keyword">async</span> (<span class="hljs-attr">req</span>: <span class="hljs-title class_">Request</span>, <span class="hljs-attr">res</span>: <span class="hljs-title class_">Response</span>) => {
<span class="hljs-keyword">const</span> { id } = req.<span class="hljs-property">params</span>; <span class="hljs-comment">// 从动态路由中提取参数</span>
<span class="hljs-keyword">const</span> data = { id }
<span class="hljs-title class_">ResponseUtil</span>.<span class="hljs-title function_">success</span>(res, data, <span class="hljs-string">"查询成功"</span>, <span class="hljs-number">20000</span>);
});
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/cc6038f562b5c04cec7cc22961b1232f.png" alt="image.png" /></p>
留言