哈喽,大家好,我是有勇气的牛排(全网同名)🐮
有问题的小伙伴欢迎在文末评论,点赞、收藏是对我最大的支持!!!。
文章目录
前言
SpringBoot是基于Spring开发的开源项目,属于快速开发框架封装了常用的依赖,能够快速整合第三方框架,简化了xml配置,并且去全部采用注解的方式,内置Tomcat、Jetty、Undertow等,默认集成SpringMVC框架。
1 创建Maven项目
maven配置
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.8.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
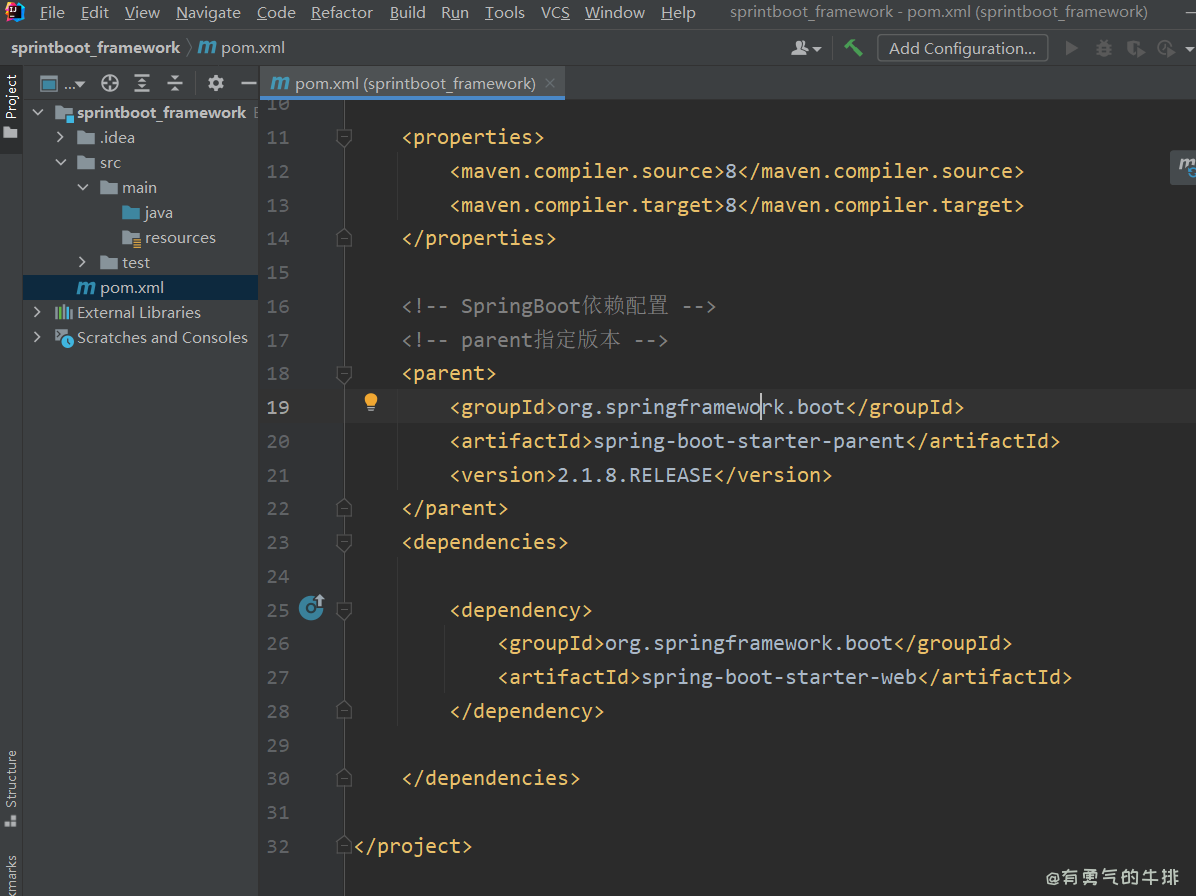
2 HelloWorld
package com.couragesteak.service;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@EnableAutoConfiguration
public class HelloWorldService {
@RequestMapping("/hello")
public String index() {
return "你好,有勇气的牛排";
}
public static void main(String[] args) {
SpringApplication.run(HelloWorldService.class, args);
}
}
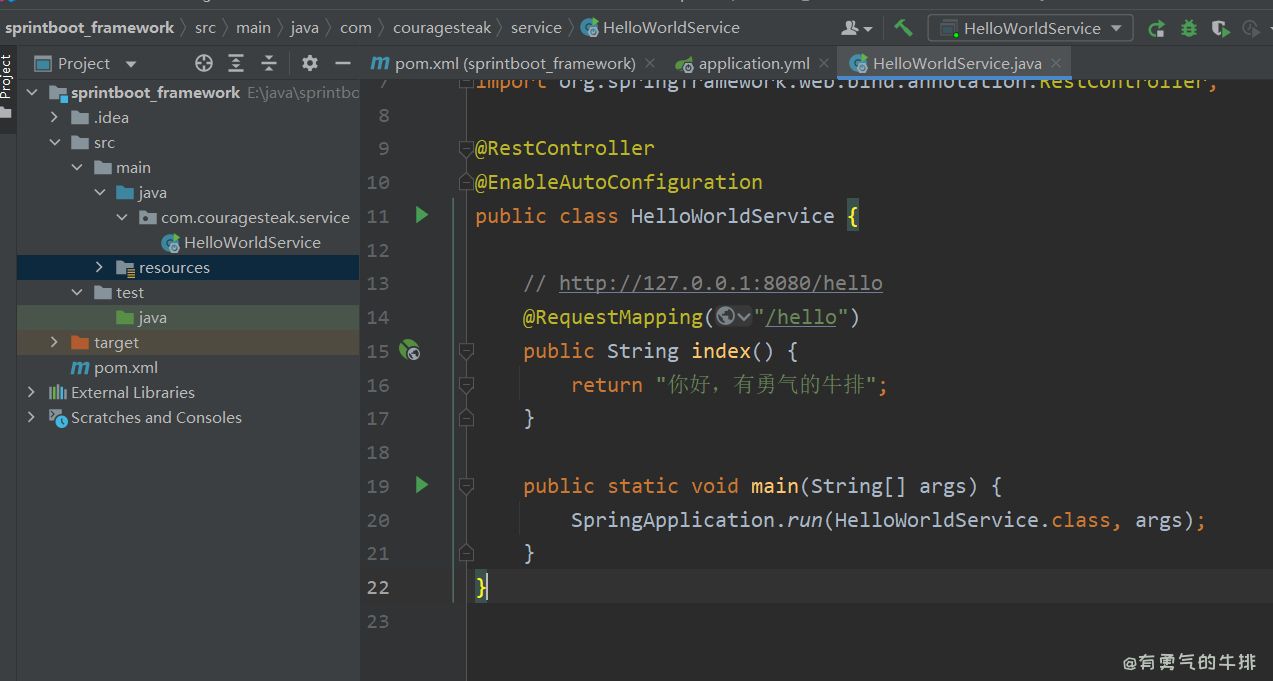
2.1 RequestController与 Controller
@RequestController
由 SpringMVC提供,相当于在每个方法添加@ResponseBody
注解。
如果在类上加 @RequestController
,该类中所有 SpringMVC接口映射均返回json格式。
2.2 主类定义 App.java
package com.couragesteak;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class App {
public static void main(String[] args) {
SpringApplication.run(App.class, args);
}
}
3 项目目录架构
com.couragesteak.controller
:视图层,web和接口(业务逻辑)
com.couragesteak.service
:业务逻辑层
com.couragesteak.dao
:数据访问层
3.1 静态资源
springboot默认静态资源目录位于 classpath(resource)下
,目录名需要符合如下规则
/static
/public
/resource
/META-INF/resources
4.2 配置文件
4.2.1 yml类型(推荐)与 properties
位置:/resource/application.yml
cs:
name: cs
star: 999
位置:/resource/application.properties
ReadConfigService.java
package com.couragesteak.service;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ReadConfigService {
@Value("${cs.name}")
private String name;
@Value("${cs.star}")
private String star;
@RequestMapping("/getProperties")
public String getProperties() {
return name + ":" + star;
}
}

4.2.2 yaml中文件占位符
在SpringBoot的配置文件中,我们可以使用SpringBoot提供的的一些随机数,来制定找不到属性时的默认值
{random.value}、{random.int}、${random.long}
{random.int(10)}、{random.int[1024,65536]}
-${app.name:默认值}
4.2.3 多环境配置
application.yml
spring:
profiles:
active: dev
application-dev.yml:开发环境
cs:
name: "有勇气的牛排"
star: 9
age: ${radom.int(10)}
spring:
http:
encoding:
force: true
charset: UTF-8
freemarker:
allow-request-override: false
cache: false
check-template-location: true
charset: UTF-8
content-type: text/html; charset=utf-8
expose-request-attributes: false
expose-session-attributes: false
expose-spring-macro-helpers: false
suffix: .ftl
template-loader-path: classpath:/templates
thymeleaf:
prefix: classpath:/templates/
check-template-location: true
cache: false
suffix: .html
encoding: UTF-8
mode: HTML5
datasource:
url: jdbc:mysql://localhost:3306/spring_boot
username: root
password: root123456
driver-class-name: com.mysql.jdbc.Driver
application-test.yml:测试环境
...
application-prd.yml:生产环境
...
4.2.4 核心配置
spring:
profiles:
active: dev
server:
port: 8081
servlet:
context-path: /
Springboot 默认的情况下整合tomcat容器,8080端口。
参考:
<p><font face="楷体,华文行楷,隶书,黑体" color="red" size="5"><strong>哈喽,大家好,我是有勇气的牛排(全网同名)🐮</strong></font></p>
<p><font face="楷体,华文行楷,隶书,黑体" color="blue" size="5"><strong>有问题的小伙伴欢迎在文末评论,点赞、收藏是对我最大的支持!!!。</strong></font></p>
<p><h3>文章目录</h3><ul><ul><li><a href="#_6">前言</a></li><li><a href="#1_Maven_11">1 创建Maven项目</a></li><li><a href="#2_HelloWorld_35">2 HelloWorld</a></li><ul><li><a href="#21_RequestController_Controller_64">2.1 RequestController与 Controller</a></li><li><a href="#22__Appjava_70">2.2 主类定义 App.java</a></li></ul><li><a href="#3__97">3 项目目录架构</a></li><ul><li><a href="#31__104">3.1 静态资源</a></li><li><a href="#42__114">4.2 配置文件</a></li><ul><li><a href="#421_yml_properties_116">4.2.1 yml类型(推荐)与 properties</a></li><li><a href="#422_yaml_165">4.2.2 yaml中文件占位符</a></li><li><a href="#423__175">4.2.3 多环境配置</a></li><li><a href="#424__249">4.2.4 核心配置</a></li></ul></ul></ul></ul></p>
<h2><a id="_6"></a>前言</h2>
<p>SpringBoot是基于Spring开发的开源项目,属于快速开发框架封装了常用的依赖,能够快速整合第三方框架,简化了xml配置,并且去全部采用注解的方式,内置Tomcat、Jetty、Undertow等,默认集成SpringMVC框架。</p>
<h2><a id="1_Maven_11"></a>1 创建Maven项目</h2>
<p>maven配置</p>
<pre><div class="hljs"><code class="lang-xml"><span class="hljs-comment"><!-- SpringBoot依赖配置 --></span>
<span class="hljs-comment"><!-- parent指定版本 --></span>
<span class="hljs-tag"><<span class="hljs-name">parent</span>></span>
<span class="hljs-tag"><<span class="hljs-name">groupId</span>></span>org.springframework.boot<span class="hljs-tag"></<span class="hljs-name">groupId</span>></span>
<span class="hljs-tag"><<span class="hljs-name">artifactId</span>></span>spring-boot-starter-parent<span class="hljs-tag"></<span class="hljs-name">artifactId</span>></span>
<span class="hljs-tag"><<span class="hljs-name">version</span>></span>2.1.8.RELEASE<span class="hljs-tag"></<span class="hljs-name">version</span>></span>
<span class="hljs-tag"></<span class="hljs-name">parent</span>></span>
<span class="hljs-tag"><<span class="hljs-name">dependencies</span>></span>
<span class="hljs-tag"><<span class="hljs-name">dependency</span>></span>
<span class="hljs-tag"><<span class="hljs-name">groupId</span>></span>org.springframework.boot<span class="hljs-tag"></<span class="hljs-name">groupId</span>></span>
<span class="hljs-tag"><<span class="hljs-name">artifactId</span>></span>spring-boot-starter-web<span class="hljs-tag"></<span class="hljs-name">artifactId</span>></span>
<span class="hljs-tag"></<span class="hljs-name">dependency</span>></span>
<span class="hljs-tag"></<span class="hljs-name">dependencies</span>></span>
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/9fd1f5c63239bc798b499b9d06b3e163.png" alt="image.png" /></p>
<h2><a id="2_HelloWorld_35"></a>2 HelloWorld</h2>
<pre><div class="hljs"><code class="lang-java"><span class="hljs-keyword">package</span> com.couragesteak.service;
<span class="hljs-keyword">import</span> org.springframework.boot.SpringApplication;
<span class="hljs-keyword">import</span> org.springframework.boot.autoconfigure.EnableAutoConfiguration;
<span class="hljs-keyword">import</span> org.springframework.web.bind.annotation.RequestMapping;
<span class="hljs-keyword">import</span> org.springframework.web.bind.annotation.RestController;
<span class="hljs-meta">@RestController</span>
<span class="hljs-meta">@EnableAutoConfiguration</span>
<span class="hljs-keyword">public</span> <span class="hljs-keyword">class</span> <span class="hljs-title class_">HelloWorldService</span> {
<span class="hljs-comment">// http://127.0.0.1:8080/hello</span>
<span class="hljs-meta">@RequestMapping("/hello")</span>
<span class="hljs-keyword">public</span> String <span class="hljs-title function_">index</span><span class="hljs-params">()</span> {
<span class="hljs-keyword">return</span> <span class="hljs-string">"你好,有勇气的牛排"</span>;
}
<span class="hljs-keyword">public</span> <span class="hljs-keyword">static</span> <span class="hljs-keyword">void</span> <span class="hljs-title function_">main</span><span class="hljs-params">(String[] args)</span> {
SpringApplication.run(HelloWorldService.class, args);
}
}
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/ba8f20620e9ece089f23798ea88a3566.png" alt="image.png" /></p>
<h3><a id="21_RequestController_Controller_64"></a>2.1 RequestController与 Controller</h3>
<p><code>@RequestController</code> 由 SpringMVC提供,相当于在每个方法添加<code>@ResponseBody</code>注解。</p>
<p>如果在类上加 <code>@RequestController</code>,该类中所有 SpringMVC接口映射均返回json格式。</p>
<h3><a id="22__Appjava_70"></a>2.2 主类定义 App.java</h3>
<pre><div class="hljs"><code class="lang-java"><span class="hljs-keyword">package</span> com.couragesteak;
<span class="hljs-comment">/*
* @Author : 有勇气的牛排
* @FileName: APP.java
* desc : 启动
* */</span>
<span class="hljs-keyword">import</span> org.springframework.boot.SpringApplication;
<span class="hljs-keyword">import</span> org.springframework.boot.autoconfigure.SpringBootApplication;
<span class="hljs-meta">@SpringBootApplication</span>
<span class="hljs-keyword">public</span> <span class="hljs-keyword">class</span> <span class="hljs-title class_">App</span> {
<span class="hljs-keyword">public</span> <span class="hljs-keyword">static</span> <span class="hljs-keyword">void</span> <span class="hljs-title function_">main</span><span class="hljs-params">(String[] args)</span> {
SpringApplication.run(App.class, args);
}
<span class="hljs-comment">/*
* @ComponentScan 扫包范围:
* 当前启动类同级包,或者子包
* */</span>
}
</code></div></pre>
<h2><a id="3__97"></a>3 项目目录架构</h2>
<p><code>com.couragesteak.controller</code>:视图层,web和接口(业务逻辑)<br />
<code>com.couragesteak.service</code>:业务逻辑层<br />
<code>com.couragesteak.dao</code>:数据访问层</p>
<h3><a id="31__104"></a>3.1 静态资源</h3>
<p>springboot默认静态资源目录位于 <code>classpath(resource)下</code>,目录名需要符合如下规则</p>
<p><code>/static</code><br />
<code>/public</code><br />
<code>/resource</code><br />
<code>/META-INF/resources</code></p>
<h3><a id="42__114"></a>4.2 配置文件</h3>
<h4><a id="421_yml_properties_116"></a>4.2.1 yml类型(推荐)与 properties</h4>
<p>位置:<code>/resource/application.yml</code></p>
<pre><div class="hljs"><code class="lang-yaml"><span class="hljs-attr">cs:</span>
<span class="hljs-attr">name:</span> <span class="hljs-string">cs</span>
<span class="hljs-attr">star:</span> <span class="hljs-number">999</span>
</code></div></pre>
<p>位置:<code>/resource/application.properties</code></p>
<pre><div class="hljs"><code class="lang-yml"><span class="hljs-comment">#cs.name=couragesteak</span>
<span class="hljs-comment">#cs.star=9</span>
</code></div></pre>
<p>ReadConfigService.java</p>
<pre><div class="hljs"><code class="lang-java"><span class="hljs-comment">/*
* @Author : 有勇气的牛排
* @FileName: ReadConfigService.java
* desc : 读取配置
* */</span>
<span class="hljs-keyword">package</span> com.couragesteak.service;
<span class="hljs-keyword">import</span> org.springframework.beans.factory.annotation.Value;
<span class="hljs-keyword">import</span> org.springframework.web.bind.annotation.RequestMapping;
<span class="hljs-keyword">import</span> org.springframework.web.bind.annotation.RestController;
<span class="hljs-meta">@RestController</span>
<span class="hljs-keyword">public</span> <span class="hljs-keyword">class</span> <span class="hljs-title class_">ReadConfigService</span> {
<span class="hljs-meta">@Value("${cs.name}")</span>
<span class="hljs-keyword">private</span> String name;
<span class="hljs-meta">@Value("${cs.star}")</span>
<span class="hljs-keyword">private</span> String star;
<span class="hljs-comment">//</span>
<span class="hljs-meta">@RequestMapping("/getProperties")</span>
<span class="hljs-keyword">public</span> String <span class="hljs-title function_">getProperties</span><span class="hljs-params">()</span> {
<span class="hljs-keyword">return</span> name + <span class="hljs-string">":"</span> + star;
}
}
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/073169f2035972c8fb9284aaa1009019.png" alt="image.png" /></p>
<h4><a id="422_yaml_165"></a>4.2.2 yaml中文件占位符</h4>
<p>在SpringBoot的配置文件中,我们可以使用SpringBoot提供的的一些随机数,来制定找不到属性时的默认值</p>
<p>{random.value}、{random.int}、${random.long}</p>
<p>{random.int(10)}、{random.int[1024,65536]}</p>
<p>-${app.name:默认值}</p>
<h4><a id="423__175"></a>4.2.3 多环境配置</h4>
<p>application.yml</p>
<pre><div class="hljs"><code class="lang-yaml"><span class="hljs-attr">spring:</span>
<span class="hljs-attr">profiles:</span>
<span class="hljs-attr">active:</span> <span class="hljs-string">dev</span>
<span class="hljs-comment"># active: test</span>
<span class="hljs-comment"># active: prd</span>
</code></div></pre>
<p>application-dev.yml:开发环境</p>
<pre><div class="hljs"><code class="lang-yaml"><span class="hljs-attr">cs:</span>
<span class="hljs-attr">name:</span> <span class="hljs-string">"有勇气的牛排"</span>
<span class="hljs-attr">star:</span> <span class="hljs-number">9</span>
<span class="hljs-attr">age:</span> <span class="hljs-string">${radom.int(10)}</span>
<span class="hljs-attr">spring:</span>
<span class="hljs-attr">http:</span>
<span class="hljs-attr">encoding:</span>
<span class="hljs-attr">force:</span> <span class="hljs-literal">true</span>
<span class="hljs-comment">### 模版引擎编码为UTF-8</span>
<span class="hljs-attr">charset:</span> <span class="hljs-string">UTF-8</span>
<span class="hljs-comment"># 模板</span>
<span class="hljs-attr">freemarker:</span>
<span class="hljs-attr">allow-request-override:</span> <span class="hljs-literal">false</span>
<span class="hljs-attr">cache:</span> <span class="hljs-literal">false</span>
<span class="hljs-attr">check-template-location:</span> <span class="hljs-literal">true</span>
<span class="hljs-attr">charset:</span> <span class="hljs-string">UTF-8</span>
<span class="hljs-attr">content-type:</span> <span class="hljs-string">text/html;</span> <span class="hljs-string">charset=utf-8</span>
<span class="hljs-attr">expose-request-attributes:</span> <span class="hljs-literal">false</span>
<span class="hljs-attr">expose-session-attributes:</span> <span class="hljs-literal">false</span>
<span class="hljs-attr">expose-spring-macro-helpers:</span> <span class="hljs-literal">false</span>
<span class="hljs-comment">## 模版文件结尾.ftl</span>
<span class="hljs-attr">suffix:</span> <span class="hljs-string">.ftl</span>
<span class="hljs-comment">## 模版文件目录</span>
<span class="hljs-attr">template-loader-path:</span> <span class="hljs-string">classpath:/templates</span>
<span class="hljs-comment"># 模板</span>
<span class="hljs-attr">thymeleaf:</span>
<span class="hljs-comment">#prefix:指定模板所在的目录</span>
<span class="hljs-attr">prefix:</span> <span class="hljs-string">classpath:/templates/</span>
<span class="hljs-comment">#check-tempate-location: 检查模板路径是否存在</span>
<span class="hljs-attr">check-template-location:</span> <span class="hljs-literal">true</span>
<span class="hljs-comment">#cache: 是否缓存,开发模式下设置为false,避免改了模板还要重启服务器,线上设置为true,可以提高性能。</span>
<span class="hljs-attr">cache:</span> <span class="hljs-literal">false</span>
<span class="hljs-attr">suffix:</span> <span class="hljs-string">.html</span>
<span class="hljs-attr">encoding:</span> <span class="hljs-string">UTF-8</span>
<span class="hljs-attr">mode:</span> <span class="hljs-string">HTML5</span>
<span class="hljs-attr">datasource:</span>
<span class="hljs-attr">url:</span> <span class="hljs-string">jdbc:mysql://localhost:3306/spring_boot</span>
<span class="hljs-attr">username:</span> <span class="hljs-string">root</span>
<span class="hljs-attr">password:</span> <span class="hljs-string">root123456</span>
<span class="hljs-attr">driver-class-name:</span> <span class="hljs-string">com.mysql.jdbc.Driver</span>
</code></div></pre>
<p>application-test.yml:测试环境</p>
<pre><div class="hljs"><code class="lang-yaml"><span class="hljs-string">...</span>
</code></div></pre>
<p>application-prd.yml:生产环境</p>
<pre><div class="hljs"><code class="lang-yaml"><span class="hljs-string">...</span>
</code></div></pre>
<h4><a id="424__249"></a>4.2.4 核心配置</h4>
<pre><div class="hljs"><code class="lang-yaml"><span class="hljs-attr">spring:</span>
<span class="hljs-attr">profiles:</span>
<span class="hljs-attr">active:</span> <span class="hljs-string">dev</span>
<span class="hljs-comment"># active: test</span>
<span class="hljs-comment"># active: prd</span>
<span class="hljs-attr">server:</span>
<span class="hljs-comment"># 端口号</span>
<span class="hljs-attr">port:</span> <span class="hljs-number">8081</span>
<span class="hljs-attr">servlet:</span>
<span class="hljs-comment"># 设置spring-boot项目访问路径</span>
<span class="hljs-attr">context-path:</span> <span class="hljs-string">/</span>
</code></div></pre>
<p>Springboot 默认的情况下整合tomcat容器,8080端口。</p>
<p>参考:</p>
<ul>
<li>余胜军</li>
</ul>
留言