1 介绍
集合类的特点:提供一种存储空间可变的存储模型,存储的数据容量可以随时发生变。
2 Collection
2.1 集合类体系结构
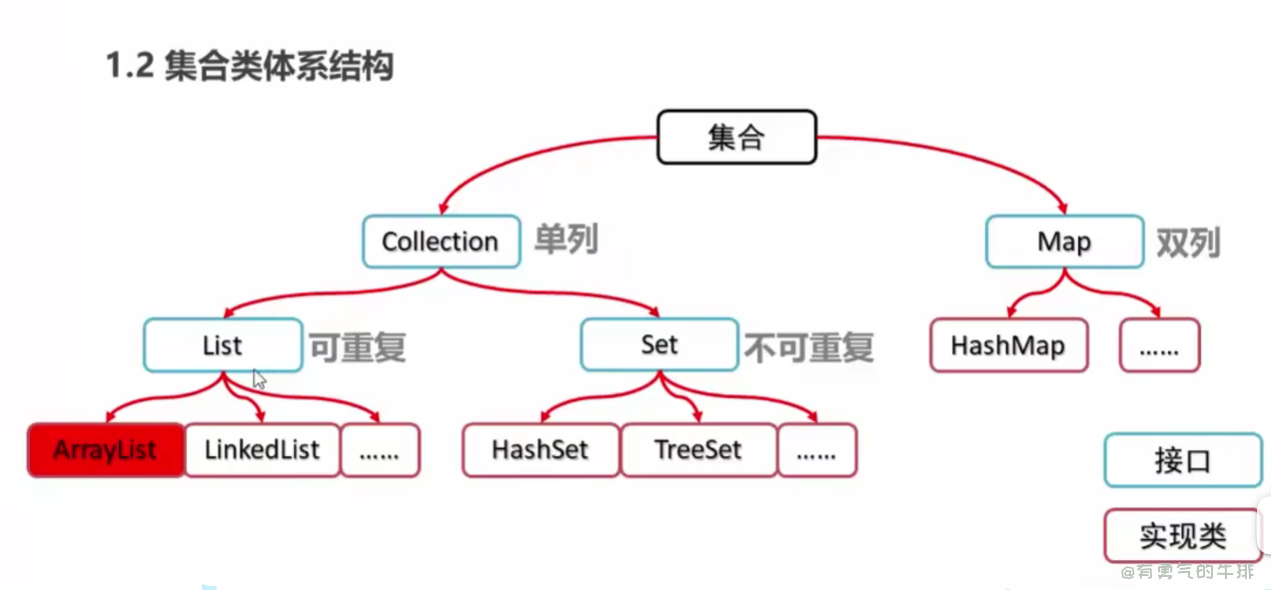
2.2 概述
软件包:java.util
-
是单列集合的顶层接口,他表示一组对象,这些对象也成为Collection的元素。
-
JDK不提供此接口的任何直接实现,他提供更具体的子接口(如Set和List)实现。
2.3 创建Collection集合的对象
import java.util.ArrayList;
import java.util.Collection;
public class CollectionDemo1 {
public static void main(String[] args) {
Collection<String> c = new ArrayList<String>();
c.add("hello");
c.add("world");
c.add("java");
System.out.println(c);
}
}
输出:
[hello, world, java]
2.4 Collection集合常用方法
boolean add(E e)
: 添加元素
boolean remove(Object o)
: 从集合中移除指定的元素。
void clear
:清空集合中的元素
boolean contains(Object o)
:判断集合中是否存在指定的元素
boolean isEmpty()
:判断集合是否为空
int size()
:集合的长度,也就是集合中元素的个数
import java.util.ArrayList;
import java.util.Collection;
public class CollectionDemo2 {
public static void main(String[] args) {
Collection<String> c = new ArrayList<String>();
System.out.println(c.add("hello"));
System.out.println(c.add("world"));
System.out.println(c.add("world"));
System.out.println(c.remove("world"));
System.out.println(c.contains("hello"));
System.out.println(c.isEmpty());
System.out.println(c.size());
System.out.println(c);
}
}
2.5 Collection集合的遍历
Iterator<E> itertor():返回此集合中元素的迭代器,通过集合的iterator() 方法得到
迭代器是通过集合的iterator方法得到的,所以我们说它是依赖于集合而存在的
Iterator中常用的方法
-
E next()
:返回迭代中的下一个元素
-
boolean hashNext()
:如果迭代具有更多元素,则返回true
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
public class ItertorDemo {
public static void main(String[] args) {
Collection<String> c = new ArrayList<String>();
c.add("hello");
c.add("world");
c.add("java");
Iterator<String> it = c.iterator();
while (it.hasNext()){
String s = it.next();
System.out.println(s);
}
}
}
2.6 Collection 集合存储学生对象并遍历
需求:创建一个存储学生对象的集合,存储3个学生对象,使用程序事项在控制台遍历该集合
思路
(1)定义学生类
(2)创建Collection集合对象
(3)创建学生对象
(4)把学生添加到集合
(5)遍历集合(迭代器方式)
学生类 Student.java
package CollectionDemo;
public class Student {
private String name;
private int age;
public Student(){}
public Student(String name, int age){
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public void setName(String name) {
this.name = name;
}
public void setAge(int age) {
this.age = age;
}
}
CollectionDemo.java
package CollectionDemo;
import java.util.ArrayDeque;
import java.util.Collection;
import java.util.Iterator;
public class CollectionDemo {
public static void main(String[] args) {
Collection<Student> c = new ArrayDeque<Student>();
Student s1 = new Student("灰太狼", 20);
Student s2 = new Student("懒羊羊", 21);
Student s3 = new Student("导演", 22);
c.add(s1);
c.add(s2);
c.add(s3);
Iterator<Student> it = c.iterator();
while (it.hasNext()) {
Student s = it.next();
System.out.println(s.getName() + "," + s.getAge());
}
}
}
输出:
灰太狼,20
懒羊羊,21
导演,22
参考地址:
https://www.bilibili.com/video/BV18J411W7cE?p=221
<h2><a id="1__0"></a>1 介绍</h2>
<p>集合类的特点:提供一种存储空间可变的存储模型,存储的数据容量可以随时发生变。</p>
<h2><a id="2_Collection_3"></a>2 Collection</h2>
<h3><a id="21__4"></a>2.1 集合类体系结构</h3>
<p><img src="https://static.couragesteak.com/article/18522612724bfdf2c3e5c4a1c4f5a986.png" alt="image.png" /></p>
<h3><a id="22__8"></a>2.2 概述</h3>
<p>软件包:java.util</p>
<ol>
<li>
<p>是单列集合的顶层接口,他表示一组对象,这些对象也成为Collection的元素。</p>
</li>
<li>
<p>JDK不提供此接口的任何直接实现,他提供更具体的子接口(如Set和List)实现。</p>
</li>
</ol>
<h3><a id="23_Collection_16"></a>2.3 创建Collection集合的对象</h3>
<ul>
<li>
<p>多态的方式</p>
</li>
<li>
<p>具体的实现类ArrayList</p>
</li>
</ul>
<pre><div class="hljs"><code class="lang-java"><span class="hljs-keyword">import</span> java.util.ArrayList;
<span class="hljs-keyword">import</span> java.util.Collection;
<span class="hljs-comment">/**
* 创建 Collection 集合的对象
* 多态的方式
* ArrayList()
* */</span>
<span class="hljs-keyword">public</span> <span class="hljs-keyword">class</span> <span class="hljs-title class_">CollectionDemo1</span> {
<span class="hljs-keyword">public</span> <span class="hljs-keyword">static</span> <span class="hljs-keyword">void</span> <span class="hljs-title function_">main</span><span class="hljs-params">(String[] args)</span> {
<span class="hljs-comment">// 创建 Collection 集合的对象</span>
Collection<String> c = <span class="hljs-keyword">new</span> <span class="hljs-title class_">ArrayList</span><String>();
<span class="hljs-comment">// 添加元素:boolean add(E e)</span>
c.add(<span class="hljs-string">"hello"</span>);
c.add(<span class="hljs-string">"world"</span>);
c.add(<span class="hljs-string">"java"</span>);
<span class="hljs-comment">// 输出集合对象</span>
System.out.println(c);
}
}
</code></div></pre>
<p>输出:</p>
<pre><div class="hljs"><code class="lang-java">[hello, world, java]
</code></div></pre>
<h3><a id="24_Collection_56"></a>2.4 Collection集合常用方法</h3>
<p><code>boolean add(E e)</code>: 添加元素</p>
<p><code>boolean remove(Object o)</code>: 从集合中移除指定的元素。</p>
<p><code>void clear</code>:清空集合中的元素</p>
<p><code>boolean contains(Object o)</code>:判断集合中是否存在指定的元素</p>
<p><code>boolean isEmpty()</code>:判断集合是否为空</p>
<p><code>int size()</code>:集合的长度,也就是集合中元素的个数</p>
<pre><div class="hljs"><code class="lang-java"><span class="hljs-keyword">import</span> java.util.ArrayList;
<span class="hljs-keyword">import</span> java.util.Collection;
<span class="hljs-comment">/**
* Collection 集合常用方法
* boolean add(E e): 添加元素
* boolean remove(Object o): 从集合中移除指定的元素。
* void clear:清空集合中的元素
* boolean contains(Object o):判断集合中是否存在指定的元素
* boolean isEmpty():判断集合是否为空
* int size()`:集合的长度,也就是集合中元素的个数
* */</span>
<span class="hljs-keyword">public</span> <span class="hljs-keyword">class</span> <span class="hljs-title class_">CollectionDemo2</span> {
<span class="hljs-keyword">public</span> <span class="hljs-keyword">static</span> <span class="hljs-keyword">void</span> <span class="hljs-title function_">main</span><span class="hljs-params">(String[] args)</span> {
<span class="hljs-comment">// 创建集合对象</span>
Collection<String> c = <span class="hljs-keyword">new</span> <span class="hljs-title class_">ArrayList</span><String>();
<span class="hljs-comment">// boolean add(E e): 添加元素</span>
System.out.println(c.add(<span class="hljs-string">"hello"</span>));
System.out.println(c.add(<span class="hljs-string">"world"</span>));
System.out.println(c.add(<span class="hljs-string">"world"</span>));
<span class="hljs-comment">// boolean remove(Object o): 从集合中移除指定的元素。</span>
<span class="hljs-comment">// 成功返回true 没有元素 返回flase</span>
System.out.println(c.remove(<span class="hljs-string">"world"</span>));
<span class="hljs-comment">// void clear:清空集合中的元素</span>
<span class="hljs-comment">// c.clear();</span>
<span class="hljs-comment">// boolean contains(Object o):判断集合中是否存在指定的元素</span>
System.out.println(c.contains(<span class="hljs-string">"hello"</span>));
<span class="hljs-comment">// boolean isEmpty():判断集合是否为空</span>
System.out.println(c.isEmpty());
<span class="hljs-comment">// int size()`:集合的长度,也就是集合中元素的个数</span>
System.out.println(c.size());
<span class="hljs-comment">// 输出集合对象</span>
System.out.println(c);
}
}
</code></div></pre>
<h3><a id="25_Collection_117"></a>2.5 Collection集合的遍历</h3>
<ul>
<li>Iterator:迭代器,集合的专用遍历方式</li>
</ul>
<p>Iterator<E> itertor():返回此集合中元素的迭代器,通过集合的iterator() 方法得到</p>
<p>迭代器是通过集合的iterator方法得到的,所以我们说它是依赖于集合而存在的</p>
<p><strong>Iterator中常用的方法</strong></p>
<ol>
<li>
<p><code>E next()</code>:返回迭代中的下一个元素</p>
</li>
<li>
<p><code>boolean hashNext()</code>:如果迭代具有更多元素,则返回true</p>
</li>
</ol>
<pre><div class="hljs"><code class="lang-java"><span class="hljs-keyword">import</span> java.util.ArrayList;
<span class="hljs-keyword">import</span> java.util.Collection;
<span class="hljs-keyword">import</span> java.util.Iterator;
<span class="hljs-keyword">public</span> <span class="hljs-keyword">class</span> <span class="hljs-title class_">ItertorDemo</span> {
<span class="hljs-keyword">public</span> <span class="hljs-keyword">static</span> <span class="hljs-keyword">void</span> <span class="hljs-title function_">main</span><span class="hljs-params">(String[] args)</span> {
<span class="hljs-comment">// 创建集合对象</span>
Collection<String> c = <span class="hljs-keyword">new</span> <span class="hljs-title class_">ArrayList</span><String>();
<span class="hljs-comment">// 添加元素</span>
c.add(<span class="hljs-string">"hello"</span>);
c.add(<span class="hljs-string">"world"</span>);
c.add(<span class="hljs-string">"java"</span>);
<span class="hljs-comment">// Iterator<E> itertor():返回此集合中元素的迭代器,通过集合的iterator() 方法得到</span>
Iterator<String> it = c.iterator();
<span class="hljs-comment">// E next():返回迭代中的下一个元素</span>
<span class="hljs-comment">// System.out.println(it.next());</span>
<span class="hljs-comment">// System.out.println(it.next());</span>
<span class="hljs-comment">// System.out.println(it.next());</span>
<span class="hljs-comment">// System.out.println(it.next()); // 元素不存在 异常</span>
<span class="hljs-comment">// 输出:</span>
<span class="hljs-comment">// hello</span>
<span class="hljs-comment">// world</span>
<span class="hljs-keyword">while</span> (it.hasNext()){
<span class="hljs-type">String</span> <span class="hljs-variable">s</span> <span class="hljs-operator">=</span> it.next();
System.out.println(s);
}
}
}
</code></div></pre>
<h3><a id="26_Collection__169"></a>2.6 Collection 集合存储学生对象并遍历</h3>
<p>需求:创建一个存储学生对象的集合,存储3个学生对象,使用程序事项在控制台遍历该集合</p>
<p><strong>思路</strong></p>
<p>(1)定义学生类</p>
<p>(2)创建Collection集合对象</p>
<p>(3)创建学生对象</p>
<p>(4)把学生添加到集合</p>
<p>(5)遍历集合(迭代器方式)</p>
<p>学生类 <code>Student.java</code></p>
<pre><div class="hljs"><code class="lang-java"><span class="hljs-keyword">package</span> CollectionDemo;
<span class="hljs-keyword">public</span> <span class="hljs-keyword">class</span> <span class="hljs-title class_">Student</span> {
<span class="hljs-keyword">private</span> String name;
<span class="hljs-keyword">private</span> <span class="hljs-type">int</span> age;
<span class="hljs-comment">// 无参构造</span>
<span class="hljs-keyword">public</span> <span class="hljs-title function_">Student</span><span class="hljs-params">()</span>{}
<span class="hljs-comment">// 带参构造</span>
<span class="hljs-keyword">public</span> <span class="hljs-title function_">Student</span><span class="hljs-params">(String name, <span class="hljs-type">int</span> age)</span>{
<span class="hljs-built_in">this</span>.name = name;
<span class="hljs-built_in">this</span>.age = age;
}
<span class="hljs-keyword">public</span> String <span class="hljs-title function_">getName</span><span class="hljs-params">()</span> {
<span class="hljs-keyword">return</span> name;
}
<span class="hljs-keyword">public</span> <span class="hljs-type">int</span> <span class="hljs-title function_">getAge</span><span class="hljs-params">()</span> {
<span class="hljs-keyword">return</span> age;
}
<span class="hljs-keyword">public</span> <span class="hljs-keyword">void</span> <span class="hljs-title function_">setName</span><span class="hljs-params">(String name)</span> {
<span class="hljs-built_in">this</span>.name = name;
}
<span class="hljs-keyword">public</span> <span class="hljs-keyword">void</span> <span class="hljs-title function_">setAge</span><span class="hljs-params">(<span class="hljs-type">int</span> age)</span> {
<span class="hljs-built_in">this</span>.age = age;
}
}
</code></div></pre>
<p><code>CollectionDemo.java</code></p>
<pre><div class="hljs"><code class="lang-java"><span class="hljs-keyword">package</span> CollectionDemo;
<span class="hljs-keyword">import</span> java.util.ArrayDeque;
<span class="hljs-keyword">import</span> java.util.Collection;
<span class="hljs-keyword">import</span> java.util.Iterator;
<span class="hljs-keyword">public</span> <span class="hljs-keyword">class</span> <span class="hljs-title class_">CollectionDemo</span> {
<span class="hljs-keyword">public</span> <span class="hljs-keyword">static</span> <span class="hljs-keyword">void</span> <span class="hljs-title function_">main</span><span class="hljs-params">(String[] args)</span> {
<span class="hljs-comment">// 创建 Collection 集合对象</span>
Collection<Student> c = <span class="hljs-keyword">new</span> <span class="hljs-title class_">ArrayDeque</span><Student>();
<span class="hljs-comment">// 创建学生对象</span>
<span class="hljs-type">Student</span> <span class="hljs-variable">s1</span> <span class="hljs-operator">=</span> <span class="hljs-keyword">new</span> <span class="hljs-title class_">Student</span>(<span class="hljs-string">"灰太狼"</span>, <span class="hljs-number">20</span>);
<span class="hljs-type">Student</span> <span class="hljs-variable">s2</span> <span class="hljs-operator">=</span> <span class="hljs-keyword">new</span> <span class="hljs-title class_">Student</span>(<span class="hljs-string">"懒羊羊"</span>, <span class="hljs-number">21</span>);
<span class="hljs-type">Student</span> <span class="hljs-variable">s3</span> <span class="hljs-operator">=</span> <span class="hljs-keyword">new</span> <span class="hljs-title class_">Student</span>(<span class="hljs-string">"导演"</span>, <span class="hljs-number">22</span>);
<span class="hljs-comment">// 把学生添加到集合</span>
c.add(s1);
c.add(s2);
c.add(s3);
<span class="hljs-comment">// 遍历集合(迭代器方式)</span>
Iterator<Student> it = c.iterator();
<span class="hljs-keyword">while</span> (it.hasNext()) {
<span class="hljs-type">Student</span> <span class="hljs-variable">s</span> <span class="hljs-operator">=</span> it.next();
System.out.println(s.getName() + <span class="hljs-string">","</span> + s.getAge());
}
}
}
</code></div></pre>
<p>输出:</p>
<pre><div class="hljs"><code class="lang-java">灰太狼,<span class="hljs-number">20</span>
懒羊羊,<span class="hljs-number">21</span>
导演,<span class="hljs-number">22</span>
</code></div></pre>
<p>参考地址:<br />
https://www.bilibili.com/video/BV18J411W7cE?p=221</p>
留言