ajax异步请求教程与案例
1 前言
AJAX(Asynchronous JavaScript and XML)是一种在不重新加载整个页面的情况下与服务器交换数据并更新部分网页的技术。它使得网页能够在后台与服务器进行异步交互,而不会影响页面的显示或用户的操作体验,从而实现更加动态和互动的网页应用。
AJAX 主要基于以下几种技术:
- JavaScript:用来处理网页的交互逻辑。
- XMLHttpRequest 对象:这是 JavaScript 提供的一个用于发送 HTTP 请求和接收响应的 API,能够实现异步通信。
- DOM(文档对象模型):用于动态更新网页内容。
- JSON 或 XML:用于在客户端和服务器之间传输数据。虽然名字里有 “XML”,但是 JSON 在实际应用中比 XML 更为常用,因其更轻量和易于解析
2 初始化
导入
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
返回数据准备
{
"code": 200,
"msg": "请求成功",
"data": {
"title": "有勇气的牛排",
"url": "https://www.couragesteak.com/",
"tag": [
"Python",
"Java"
],
"data": [
{
"id": 1,
"title": "Java教程"
},
{
"id": 2,
"title": "Python人工智能"
}
]
}
}
3 ajax完整请求结构
$.ajax({
url: '/test',
type: 'POST',
dataType: 'json',
beforeSend: function (request) {
},
headers: {
'Authorization': "token"
},
data: {
'money': money
},
success:function (res) {
},
error:function () {
}
});
4 GET 请求
<button id="getBtn">GET请求数据</button>
<script>
async function get_data() {
try {
const res = await $.ajax({
url: 'http://127.0.0.1:8090/test_json',
type: 'GET',
dataType: 'json',
});
console.log(">>> 请求success");
console.log(res);
return res.data;
} catch (error) {
console.log(">>> 请求error");
console.log(error);
}
}
$('#getBtn').on('click', async function () {
const data = await get_data();
console.log(">>> 最终结果");
console.log(data);
console.log(data.title);
});
</script>
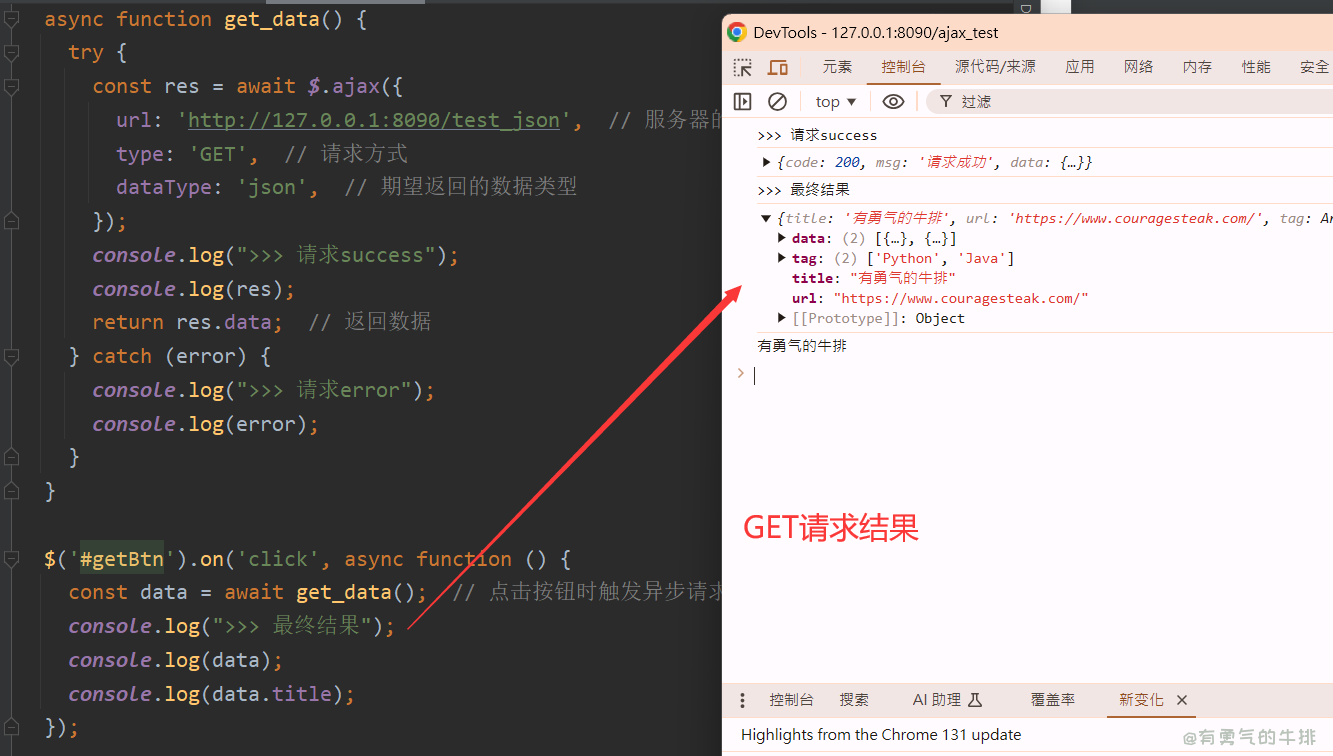
5 post json 案例
json这里指json请求体
<script>
async function post_data(data) {
try {
const res = await $.ajax({
url: 'http://127.0.0.1:8090/test_json_post',
type: 'POST',
contentType: 'application/json',
dataType: 'json',
data: JSON.stringify(data),
});
console.log(">>> 请求success");
console.log(res);
return res;
} catch (error) {
console.log(">>> 请求error");
console.log(error);
}
}
$('#postBtn').on('click', async function () {
const dataToSend = {
nickname: '有勇气的牛排'
};
const data2 = await post_data(dataToSend);
console.log(">>> 最终结果");
console.log(data2);
});
</script>
6 通用请求封装
<script>
async function ajaxRequest({url, method = 'GET', data = null, contentType = 'application/json', dataType = 'json'}) {
try {
const options = {
url: url,
type: method,
contentType: contentType,
dataType: dataType,
};
if (method === 'POST' && data) {
options.data = JSON.stringify(data);
} else if (method === 'GET' && data) {
options.data = data;
}
const res = await $.ajax(options);
console.log(">>> 请求成功");
console.log(res);
return res.data;
} catch (error) {
console.log(">>> 请求失败");
console.log(error);
}
}
$(document).ready(function () {
$('#getBtn').on('click', async function () {
const data = await ajaxRequest({
url: 'http://127.0.0.1:8090/test_json',
method: 'GET',
});
console.log(">>> GET 请求结果");
console.log(data);
});
$('#postBtn').on('click', async function () {
const dataToSend = {
nickname: '有勇气的牛排'
};
const data = await ajaxRequest({
url: 'http://127.0.0.1:8090/test_json_post',
method: 'POST',
data: dataToSend,
});
console.log(">>> POST 请求结果");
console.log(data);
});
});
</script>
<h1><a id="ajax_0"></a>ajax异步请求教程与案例</h1>
<h2><a id="1__2"></a>1 前言</h2>
<p>AJAX(Asynchronous JavaScript and XML)是一种在不重新加载整个页面的情况下与服务器交换数据并更新部分网页的技术。它使得网页能够在后台与服务器进行异步交互,而不会影响页面的显示或用户的操作体验,从而实现更加动态和互动的网页应用。</p>
<p>AJAX 主要基于以下几种技术:</p>
<ol>
<li><strong>JavaScript</strong>:用来处理网页的交互逻辑。</li>
<li><strong>XMLHttpRequest 对象</strong>:这是 JavaScript 提供的一个用于发送 HTTP 请求和接收响应的 API,能够实现异步通信。</li>
<li><strong>DOM(文档对象模型)</strong>:用于动态更新网页内容。</li>
<li><strong>JSON 或 XML</strong>:用于在客户端和服务器之间传输数据。虽然名字里有 “XML”,但是 JSON 在实际应用中比 XML 更为常用,因其更轻量和易于解析</li>
</ol>
<h2><a id="2__13"></a>2 初始化</h2>
<p>导入</p>
<pre><div class="hljs"><code class="lang-shell"><script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</code></div></pre>
<p>返回数据准备</p>
<pre><div class="hljs"><code class="lang-json"><span class="hljs-punctuation">{</span>
<span class="hljs-attr">"code"</span><span class="hljs-punctuation">:</span> <span class="hljs-number">200</span><span class="hljs-punctuation">,</span>
<span class="hljs-attr">"msg"</span><span class="hljs-punctuation">:</span> <span class="hljs-string">"请求成功"</span><span class="hljs-punctuation">,</span>
<span class="hljs-attr">"data"</span><span class="hljs-punctuation">:</span> <span class="hljs-punctuation">{</span>
<span class="hljs-attr">"title"</span><span class="hljs-punctuation">:</span> <span class="hljs-string">"有勇气的牛排"</span><span class="hljs-punctuation">,</span>
<span class="hljs-attr">"url"</span><span class="hljs-punctuation">:</span> <span class="hljs-string">"https://www.couragesteak.com/"</span><span class="hljs-punctuation">,</span>
<span class="hljs-attr">"tag"</span><span class="hljs-punctuation">:</span> <span class="hljs-punctuation">[</span>
<span class="hljs-string">"Python"</span><span class="hljs-punctuation">,</span>
<span class="hljs-string">"Java"</span>
<span class="hljs-punctuation">]</span><span class="hljs-punctuation">,</span>
<span class="hljs-attr">"data"</span><span class="hljs-punctuation">:</span> <span class="hljs-punctuation">[</span>
<span class="hljs-punctuation">{</span>
<span class="hljs-attr">"id"</span><span class="hljs-punctuation">:</span> <span class="hljs-number">1</span><span class="hljs-punctuation">,</span>
<span class="hljs-attr">"title"</span><span class="hljs-punctuation">:</span> <span class="hljs-string">"Java教程"</span>
<span class="hljs-punctuation">}</span><span class="hljs-punctuation">,</span>
<span class="hljs-punctuation">{</span>
<span class="hljs-attr">"id"</span><span class="hljs-punctuation">:</span> <span class="hljs-number">2</span><span class="hljs-punctuation">,</span>
<span class="hljs-attr">"title"</span><span class="hljs-punctuation">:</span> <span class="hljs-string">"Python人工智能"</span>
<span class="hljs-punctuation">}</span>
<span class="hljs-punctuation">]</span>
<span class="hljs-punctuation">}</span>
<span class="hljs-punctuation">}</span>
</code></div></pre>
<h2><a id="3_ajax_48"></a>3 ajax完整请求结构</h2>
<pre><div class="hljs"><code class="lang-javascript">$.<span class="hljs-title function_">ajax</span>({
<span class="hljs-attr">url</span>: <span class="hljs-string">'/test'</span>,
<span class="hljs-attr">type</span>: <span class="hljs-string">'POST'</span>,
<span class="hljs-attr">dataType</span>: <span class="hljs-string">'json'</span>,
<span class="hljs-attr">beforeSend</span>: <span class="hljs-keyword">function</span> (<span class="hljs-params">request</span>) {
<span class="hljs-comment">// request.setRequestHeader("Authorization", "token");</span>
},
<span class="hljs-attr">headers</span>: {
<span class="hljs-string">'Authorization'</span>: <span class="hljs-string">"token"</span>
},
<span class="hljs-attr">data</span>: {
<span class="hljs-string">'money'</span>: money
},
<span class="hljs-attr">success</span>:<span class="hljs-keyword">function</span> (<span class="hljs-params">res</span>) {
},
<span class="hljs-attr">error</span>:<span class="hljs-keyword">function</span> (<span class="hljs-params"></span>) {
}
});
</code></div></pre>
<h2><a id="4_GET__73"></a>4 GET 请求</h2>
<pre><div class="hljs"><code class="lang-html"><span class="hljs-tag"><<span class="hljs-name">button</span> <span class="hljs-attr">id</span>=<span class="hljs-string">"getBtn"</span>></span>GET请求数据<span class="hljs-tag"></<span class="hljs-name">button</span>></span>
</code></div></pre>
<pre><div class="hljs"><code class="lang-javascript"><script>
<span class="hljs-keyword">async</span> <span class="hljs-keyword">function</span> <span class="hljs-title function_">get_data</span>(<span class="hljs-params"></span>) {
<span class="hljs-keyword">try</span> {
<span class="hljs-keyword">const</span> res = <span class="hljs-keyword">await</span> $.<span class="hljs-title function_">ajax</span>({
<span class="hljs-attr">url</span>: <span class="hljs-string">'http://127.0.0.1:8090/test_json'</span>, <span class="hljs-comment">// 服务器的 API 接口</span>
<span class="hljs-attr">type</span>: <span class="hljs-string">'GET'</span>, <span class="hljs-comment">// 请求方式</span>
<span class="hljs-attr">dataType</span>: <span class="hljs-string">'json'</span>, <span class="hljs-comment">// 期望返回的数据类型</span>
});
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">">>> 请求success"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(res);
<span class="hljs-keyword">return</span> res.<span class="hljs-property">data</span>; <span class="hljs-comment">// 返回数据</span>
} <span class="hljs-keyword">catch</span> (error) {
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">">>> 请求error"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(error);
}
}
$(<span class="hljs-string">'#getBtn'</span>).<span class="hljs-title function_">on</span>(<span class="hljs-string">'click'</span>, <span class="hljs-keyword">async</span> <span class="hljs-keyword">function</span> (<span class="hljs-params"></span>) {
<span class="hljs-keyword">const</span> data = <span class="hljs-keyword">await</span> <span class="hljs-title function_">get_data</span>(); <span class="hljs-comment">// 点击按钮时触发异步请求</span>
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">">>> 最终结果"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(data);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(data.<span class="hljs-property">title</span>);
});
</script>
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/69bf338360d14468bc35ddd1d00d536b.png" alt="ajax GET请求案例" /></p>
<h2><a id="5_post_json__109"></a>5 post json 案例</h2>
<p>json这里指json请求体</p>
<pre><div class="hljs"><code class="lang-javascript"><script>
<span class="hljs-keyword">async</span> <span class="hljs-keyword">function</span> <span class="hljs-title function_">post_data</span>(<span class="hljs-params">data</span>) {
<span class="hljs-keyword">try</span> {
<span class="hljs-keyword">const</span> res = <span class="hljs-keyword">await</span> $.<span class="hljs-title function_">ajax</span>({
<span class="hljs-attr">url</span>: <span class="hljs-string">'http://127.0.0.1:8090/test_json_post'</span>,
<span class="hljs-attr">type</span>: <span class="hljs-string">'POST'</span>,
<span class="hljs-attr">contentType</span>: <span class="hljs-string">'application/json'</span>,
<span class="hljs-attr">dataType</span>: <span class="hljs-string">'json'</span>,
<span class="hljs-attr">data</span>: <span class="hljs-title class_">JSON</span>.<span class="hljs-title function_">stringify</span>(data), <span class="hljs-comment">// 将传入的数据对象转换为 JSON 字符串</span>
});
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">">>> 请求success"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(res);
<span class="hljs-keyword">return</span> res;
} <span class="hljs-keyword">catch</span> (error) {
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">">>> 请求error"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(error);
}
}
$(<span class="hljs-string">'#postBtn'</span>).<span class="hljs-title function_">on</span>(<span class="hljs-string">'click'</span>, <span class="hljs-keyword">async</span> <span class="hljs-keyword">function</span> (<span class="hljs-params"></span>) {
<span class="hljs-keyword">const</span> dataToSend = {
<span class="hljs-attr">nickname</span>: <span class="hljs-string">'有勇气的牛排'</span>
};
<span class="hljs-keyword">const</span> data2 = <span class="hljs-keyword">await</span> <span class="hljs-title function_">post_data</span>(dataToSend);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">">>> 最终结果"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(data2);
});
</script>
</code></div></pre>
<h2><a id="6__146"></a>6 通用请求封装</h2>
<pre><div class="hljs"><code class="lang-javascript"><script>
<span class="hljs-comment">// 通用的 AJAX 请求函数</span>
<span class="hljs-keyword">async</span> <span class="hljs-keyword">function</span> <span class="hljs-title function_">ajaxRequest</span>(<span class="hljs-params">{url, method = <span class="hljs-string">'GET'</span>, data = <span class="hljs-literal">null</span>, contentType = <span class="hljs-string">'application/json'</span>, dataType = <span class="hljs-string">'json'</span>}</span>) {
<span class="hljs-keyword">try</span> {
<span class="hljs-keyword">const</span> options = {
<span class="hljs-attr">url</span>: url,
<span class="hljs-attr">type</span>: method, <span class="hljs-comment">// 请求方式 GET 或 POST</span>
<span class="hljs-attr">contentType</span>: contentType, <span class="hljs-comment">// 请求头,默认是 application/json</span>
<span class="hljs-attr">dataType</span>: dataType, <span class="hljs-comment">// 期望返回的数据类型</span>
};
<span class="hljs-keyword">if</span> (method === <span class="hljs-string">'POST'</span> && data) {
<span class="hljs-comment">// 如果是 POST 请求,传递 JSON 数据</span>
options.<span class="hljs-property">data</span> = <span class="hljs-title class_">JSON</span>.<span class="hljs-title function_">stringify</span>(data);
} <span class="hljs-keyword">else</span> <span class="hljs-keyword">if</span> (method === <span class="hljs-string">'GET'</span> && data) {
<span class="hljs-comment">// 如果是 GET 请求,直接传递参数对象</span>
options.<span class="hljs-property">data</span> = data;
}
<span class="hljs-keyword">const</span> res = <span class="hljs-keyword">await</span> $.<span class="hljs-title function_">ajax</span>(options); <span class="hljs-comment">// 发起 AJAX 请求</span>
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">">>> 请求成功"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(res);
<span class="hljs-keyword">return</span> res.<span class="hljs-property">data</span>; <span class="hljs-comment">// 返回服务器响应的数据</span>
} <span class="hljs-keyword">catch</span> (error) {
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">">>> 请求失败"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(error);
}
}
<span class="hljs-comment">// 页面加载完后绑定按钮事件</span>
$(<span class="hljs-variable language_">document</span>).<span class="hljs-title function_">ready</span>(<span class="hljs-keyword">function</span> (<span class="hljs-params"></span>) {
<span class="hljs-comment">// 处理 GET 请求</span>
$(<span class="hljs-string">'#getBtn'</span>).<span class="hljs-title function_">on</span>(<span class="hljs-string">'click'</span>, <span class="hljs-keyword">async</span> <span class="hljs-keyword">function</span> (<span class="hljs-params"></span>) {
<span class="hljs-keyword">const</span> data = <span class="hljs-keyword">await</span> <span class="hljs-title function_">ajaxRequest</span>({
<span class="hljs-attr">url</span>: <span class="hljs-string">'http://127.0.0.1:8090/test_json'</span>,
<span class="hljs-attr">method</span>: <span class="hljs-string">'GET'</span>, <span class="hljs-comment">// GET 请求</span>
});
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">">>> GET 请求结果"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(data);
});
<span class="hljs-comment">// 处理 POST 请求</span>
$(<span class="hljs-string">'#postBtn'</span>).<span class="hljs-title function_">on</span>(<span class="hljs-string">'click'</span>, <span class="hljs-keyword">async</span> <span class="hljs-keyword">function</span> (<span class="hljs-params"></span>) {
<span class="hljs-keyword">const</span> dataToSend = {
<span class="hljs-attr">nickname</span>: <span class="hljs-string">'有勇气的牛排'</span>
};
<span class="hljs-keyword">const</span> data = <span class="hljs-keyword">await</span> <span class="hljs-title function_">ajaxRequest</span>({
<span class="hljs-attr">url</span>: <span class="hljs-string">'http://127.0.0.1:8090/test_json_post'</span>,
<span class="hljs-attr">method</span>: <span class="hljs-string">'POST'</span>, <span class="hljs-comment">// POST 请求</span>
<span class="hljs-attr">data</span>: dataToSend,
});
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">">>> POST 请求结果"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(data);
});
});
</script>
</code></div></pre>
留言