1 前言
在前端开发中,操作 HTML 元素的样式与属性是常见的任务,无论是动态调整页面样式,还是获取元素属性进行逻辑判断,都需要熟练掌握相关技巧。JavaScript 提供了丰富的 API,允许开发者轻松获取和修改 HTML 元素的样式与属性,从而实现动态和交互式的网页效果。
本篇文章将详细讲解如何使用 JavaScript 获取 HTML 元素的样式和属性,并结合实际案例帮助您快速上手,提升开发效率。无论您是前端新手还是资深开发者,都可以从中找到实用的技巧和灵感。
2 基础样式代码
<style>
button {
overflow: hidden;
padding: 5px 10px;
border: none;
border-radius: 10vmin;
background-image: linear-gradient(90deg, #0acffe, #495aff);
color: #fff;
font-size: 55px;
font-weight: bold;
letter-spacing: 1vmin;
cursor: pointer;
width: 200px;
position: relative;
}
</style>
<button id="btn">按钮</button>
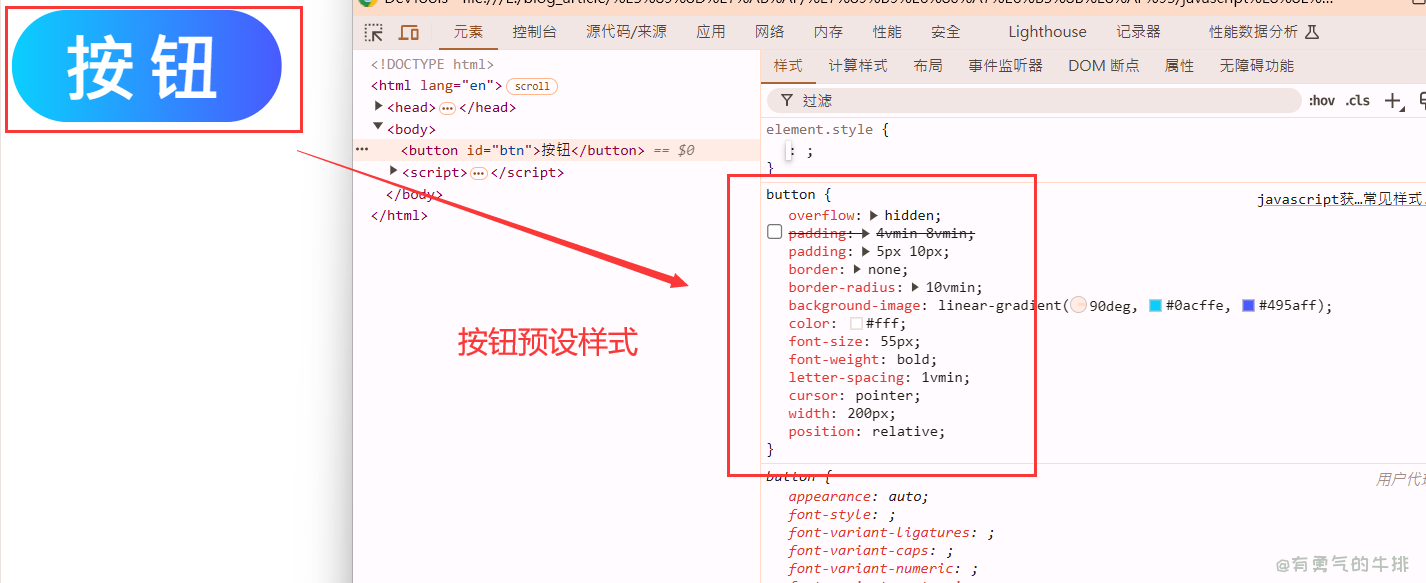
3 js提取属性案例
<script>
function getAllStylesById(elementId) {
let element = document.getElementById(elementId);
console.log("999")
console.log(element)
if (element == null) {
element = document.getElementsByTagName(elementId)
console.log(element)
}
if (!element) {
return null;
}
const computedStyle = window.getComputedStyle(element);
const styles = {};
const styles2 = {};
for (let i = 0; i < computedStyle.length; i++) {
const property = computedStyle[i];
styles2[property] = computedStyle.getPropertyValue(property);
}
console.log(styles2);
const rect = element.getBoundingClientRect();
styles["url"] = "https://www.couragesteak.com/"
styles["width"] = computedStyle.getPropertyValue("width")
styles["height"] = computedStyle.getPropertyValue("height")
styles["backgroundColor"] = computedStyle.getPropertyValue("background-color")
styles["backgroundImage"] = computedStyle.getPropertyValue("background-image")
styles["color"] = computedStyle.getPropertyValue("color")
styles["bottom"] = computedStyle.getPropertyValue("bottom")
styles["top"] = computedStyle.getPropertyValue("top")
styles["left"] = computedStyle.getPropertyValue("left")
styles["right"] = computedStyle.getPropertyValue("right")
styles["position"] = {
top: rect.top + window.scrollY,
left: rect.left + window.scrollX,
bottom: rect.bottom + window.scrollY,
right: rect.right + window.scrollX
}
return styles;
}
const styles = getAllStylesById("btn");
console.log("js获取button样式");
console.log(styles);
</script>
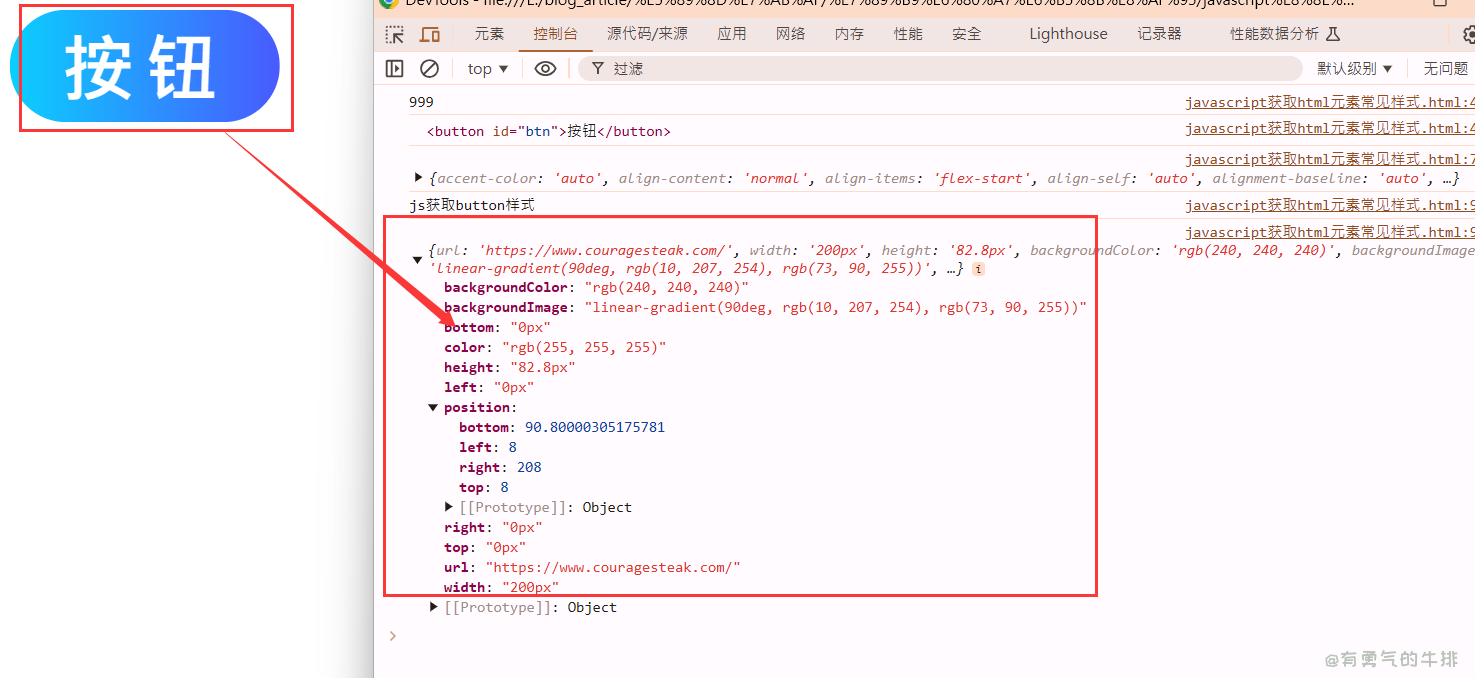
<h2><a id="1__0"></a>1 前言</h2>
<p>在前端开发中,操作 HTML 元素的样式与属性是常见的任务,无论是动态调整页面样式,还是获取元素属性进行逻辑判断,都需要熟练掌握相关技巧。JavaScript 提供了丰富的 API,允许开发者轻松获取和修改 HTML 元素的样式与属性,从而实现动态和交互式的网页效果。</p>
<p>本篇文章将详细讲解如何使用 JavaScript 获取 HTML 元素的样式和属性,并结合实际案例帮助您快速上手,提升开发效率。无论您是前端新手还是资深开发者,都可以从中找到实用的技巧和灵感。</p>
<h2><a id="2__6"></a>2 基础样式代码</h2>
<pre><div class="hljs"><code class="lang-html"><span class="hljs-tag"><<span class="hljs-name">style</span>></span><span class="language-css">
<span class="hljs-selector-tag">button</span> {
<span class="hljs-comment">/* 超出按钮部分隐藏 */</span>
<span class="hljs-attribute">overflow</span>: hidden;
<span class="hljs-comment">/* padding 撑开按钮 */</span>
<span class="hljs-comment">/*padding: 4vmin 8vmin;*/</span>
<span class="hljs-attribute">padding</span>: <span class="hljs-number">5px</span> <span class="hljs-number">10px</span>;
<span class="hljs-comment">/* 去掉按钮边框、给个圆角 */</span>
<span class="hljs-attribute">border</span>: none;
<span class="hljs-attribute">border-radius</span>: <span class="hljs-number">10vmin</span>;
<span class="hljs-comment">/* 按钮的渐变背景颜色 */</span>
<span class="hljs-attribute">background-image</span>: <span class="hljs-built_in">linear-gradient</span>(<span class="hljs-number">90deg</span>, <span class="hljs-number">#0acffe</span>, <span class="hljs-number">#495aff</span>);
<span class="hljs-comment">/* 文字颜色、大小、粗细、字间距 */</span>
<span class="hljs-attribute">color</span>: <span class="hljs-number">#fff</span>;
<span class="hljs-attribute">font-size</span>: <span class="hljs-number">55px</span>;
<span class="hljs-attribute">font-weight</span>: bold;
<span class="hljs-attribute">letter-spacing</span>: <span class="hljs-number">1vmin</span>;
<span class="hljs-comment">/* 鼠标小手 */</span>
<span class="hljs-attribute">cursor</span>: pointer;
<span class="hljs-attribute">width</span>: <span class="hljs-number">200px</span>;
<span class="hljs-attribute">position</span>: relative;
}
</span><span class="hljs-tag"></<span class="hljs-name">style</span>></span>
</code></div></pre>
<pre><div class="hljs"><code class="lang-html"><span class="hljs-tag"><<span class="hljs-name">button</span> <span class="hljs-attr">id</span>=<span class="hljs-string">"btn"</span>></span>按钮<span class="hljs-tag"></<span class="hljs-name">button</span>></span>
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/d068e9c456ade3305b6c20e76573de90.png" alt="html前端button按钮样式" /></p>
<h2><a id="3_js_43"></a>3 js提取属性案例</h2>
<pre><div class="hljs"><code class="lang-javascript"><script>
<span class="hljs-comment">/**
* 有勇气的牛排
* 获取指定id元素的所有样式,并以字典形式返回
* <span class="hljs-doctag">@param</span> {<span class="hljs-type">string</span>} <span class="hljs-variable">elementId</span> - 元素的id
* <span class="hljs-doctag">@returns</span> {<span class="hljs-type">Object | null</span>} - 返回样式字典,如果元素不存在,返回null
*/</span>
<span class="hljs-keyword">function</span> <span class="hljs-title function_">getAllStylesById</span>(<span class="hljs-params">elementId</span>) {
<span class="hljs-comment">// 获取指定id的元素</span>
<span class="hljs-keyword">let</span> element = <span class="hljs-variable language_">document</span>.<span class="hljs-title function_">getElementById</span>(elementId);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">"999"</span>)
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(element)
<span class="hljs-comment">// 检查元素是否存在</span>
<span class="hljs-keyword">if</span> (element == <span class="hljs-literal">null</span>) {
element = <span class="hljs-variable language_">document</span>.<span class="hljs-title function_">getElementsByTagName</span>(elementId)
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(element)
}
<span class="hljs-keyword">if</span> (!element) {
<span class="hljs-comment">// console.warn(`Element with id "${elementId}" not found.`);</span>
<span class="hljs-keyword">return</span> <span class="hljs-literal">null</span>;
}
<span class="hljs-comment">// 获取元素的计算样式</span>
<span class="hljs-keyword">const</span> computedStyle = <span class="hljs-variable language_">window</span>.<span class="hljs-title function_">getComputedStyle</span>(element);
<span class="hljs-keyword">const</span> styles = {};
<span class="hljs-keyword">const</span> styles2 = {};
<span class="hljs-comment">// 将所有样式属性及其值添加到字典中</span>
<span class="hljs-keyword">for</span> (<span class="hljs-keyword">let</span> i = <span class="hljs-number">0</span>; i < computedStyle.<span class="hljs-property">length</span>; i++) {
<span class="hljs-keyword">const</span> property = computedStyle[i];
styles2[property] = computedStyle.<span class="hljs-title function_">getPropertyValue</span>(property);
}
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(styles2);
<span class="hljs-comment">// 获取元素的边界矩形</span>
<span class="hljs-keyword">const</span> rect = element.<span class="hljs-title function_">getBoundingClientRect</span>();
styles[<span class="hljs-string">"url"</span>] = <span class="hljs-string">"https://www.couragesteak.com/"</span>
styles[<span class="hljs-string">"width"</span>] = computedStyle.<span class="hljs-title function_">getPropertyValue</span>(<span class="hljs-string">"width"</span>)
styles[<span class="hljs-string">"height"</span>] = computedStyle.<span class="hljs-title function_">getPropertyValue</span>(<span class="hljs-string">"height"</span>)
styles[<span class="hljs-string">"backgroundColor"</span>] = computedStyle.<span class="hljs-title function_">getPropertyValue</span>(<span class="hljs-string">"background-color"</span>)
styles[<span class="hljs-string">"backgroundImage"</span>] = computedStyle.<span class="hljs-title function_">getPropertyValue</span>(<span class="hljs-string">"background-image"</span>)
styles[<span class="hljs-string">"color"</span>] = computedStyle.<span class="hljs-title function_">getPropertyValue</span>(<span class="hljs-string">"color"</span>)
styles[<span class="hljs-string">"bottom"</span>] = computedStyle.<span class="hljs-title function_">getPropertyValue</span>(<span class="hljs-string">"bottom"</span>)
styles[<span class="hljs-string">"top"</span>] = computedStyle.<span class="hljs-title function_">getPropertyValue</span>(<span class="hljs-string">"top"</span>)
styles[<span class="hljs-string">"left"</span>] = computedStyle.<span class="hljs-title function_">getPropertyValue</span>(<span class="hljs-string">"left"</span>)
styles[<span class="hljs-string">"right"</span>] = computedStyle.<span class="hljs-title function_">getPropertyValue</span>(<span class="hljs-string">"right"</span>)
<span class="hljs-comment">// 位置</span>
styles[<span class="hljs-string">"position"</span>] = {
<span class="hljs-attr">top</span>: rect.<span class="hljs-property">top</span> + <span class="hljs-variable language_">window</span>.<span class="hljs-property">scrollY</span>, <span class="hljs-comment">// 相对于页面顶部的绝对位置</span>
<span class="hljs-attr">left</span>: rect.<span class="hljs-property">left</span> + <span class="hljs-variable language_">window</span>.<span class="hljs-property">scrollX</span>, <span class="hljs-comment">// 相对于页面左侧的绝对位置</span>
<span class="hljs-attr">bottom</span>: rect.<span class="hljs-property">bottom</span> + <span class="hljs-variable language_">window</span>.<span class="hljs-property">scrollY</span>, <span class="hljs-comment">// 相对于页面底部的绝对位置</span>
<span class="hljs-attr">right</span>: rect.<span class="hljs-property">right</span> + <span class="hljs-variable language_">window</span>.<span class="hljs-property">scrollX</span> <span class="hljs-comment">// 相对于页面右侧的绝对位置</span>
}
<span class="hljs-keyword">return</span> styles;
}
<span class="hljs-comment">// 案例</span>
<span class="hljs-keyword">const</span> styles = <span class="hljs-title function_">getAllStylesById</span>(<span class="hljs-string">"btn"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(<span class="hljs-string">"js获取button样式"</span>);
<span class="hljs-variable language_">console</span>.<span class="hljs-title function_">log</span>(styles);
</script>
</code></div></pre>
<p><img src="https://static.couragesteak.com/article/7a46c66a89e302a7e41bc9a9cc13f9a3.png" alt="image.png" /></p>
留言